import pandas as pd # 定义标背景框色函数 def background_color(val): color = 'red' if val < 60 else 'yellow' if val < 80 else 'green' return { 'background-color': color, 'border': '1px solid black' } # 创建DataFrame df = pd.DataFrame({ 'name': ['Alice', 'Bob', 'Charlie', 'David'], 'score': [70, 55, 85, 90] }) # 创建Styler对象并应用标背景框色函数 styler = df.style.applymap(background_color, subset=['score']) styler too many values to unpack (expected 2)
时间: 2023-08-18 09:08:00 浏览: 109
这个错误是因为在执行`background_color`函数时返回的字典格式不正确。`background_color`函数应该返回一个包含两个元素的元组,第一个元素是字符串格式的CSS样式,第二个元素是None。具体来说,你需要将函数的返回值改为`return ('background-color: {}; border: 1px solid black'.format(color), None)`。修改后的代码如下:
```python
import pandas as pd
# 定义标背景框色函数
def background_color(val):
color = 'red' if val < 60 else 'yellow' if val < 80 else 'green'
return ('background-color: {}; border: 1px solid black'.format(color), None)
# 创建DataFrame
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'David'],
'score': [70, 55, 85, 90]
})
# 创建Styler对象并应用标背景框色函数
styler = df.style.applymap(background_color, subset=['score'])
styler
```
这样就可以正确执行了。
相关问题
def cal_linear(iaqi_lo, iaqi_hi, bp_lo, bp_hi, cp): """ 范围缩放 """ iaqi = (iaqi_hi - iaqi_lo) * (cp - bp_lo) / (bp_hi - bp_lo) + iaqi_lo return iaqi def cal_pm25_iaqi(pm25_val): """ PM2.5的iaqi计算函数 """ if 0 <= pm25_val < 36: iaqi1 = cal_linear(0, 50, 0, 35, pm25_val) elif 36 <= pm25_val < 76: iaqi1 = cal_linear(50, 100, 35, 75, pm25_val) elif 76 <= pm25_val < 116: iaqi1 = cal_linear(100, 150, 75, 115, pm25_val) elif 116 <= pm25_val < 151: iaqi1 = cal_linear(150, 200, 115, 150, pm25_val) elif 151 <= pm25_val < 251: iaqi1 = cal_linear(200, 300, 150, 250, pm25_val) elif 251 <= pm25_val < 351: iaqi1 = cal_linear(300, 400, 250, 350, pm25_val) elif 351 <= pm25_val < 501: iaqi1 = cal_linear(400, 500, 350, 500, pm25_val) return iaqi1 def cal_pm10_iaqi(pm10_val): """ CO的iaqi计算函数 """ if 0 <= pm10_val < 51: iaqi2 = cal_linear(0, 50, 0, 50, pm10_val) elif 51 <= pm10_val < 151: iaqi2 = cal_linear(50, 100, 50, 150, pm10_val) elif 151 <= pm10_val < 251: iaqi2 = cal_linear(100, 150, 150, 250, pm10_val) elif 251 <= pm10_val < 351: iaqi2 = cal_linear(150, 200, 250, 350, pm10_val) elif 351 <= pm10_val < 421: iaqi2 = cal_linear(200, 300, 350, 420, pm10_val) elif 421 <= pm10_val < 501: iaqi2 = cal_linear(300, 400, 420, 500, pm10_val) elif 501 <= pm10_val < 601: iaqi2 = cal_linear(400, 500, 500, 600, pm10_val) return iaqi2 pm25_value = dust_weather_data['pm2.5'] pm10_value = dust_weather_data['pm10'] pm25_iaqi = cal_pm25_iaqi(pm25_value) pm10_iaqi = cal_pm10_iaqi(pm10_val) aqi = max(iaqi1,iaqi2)出错,The truth value of a Series is ambiguous. Use a.empty, a.bool(), a.item(), a.any() or a.all().
在你的代码中,出现了相同的错误。这是因为在计算aqi时,你尝试将Series对象(iaqi1和iaqi2)与标量值进行比较,导致了"The truth value of a Series is ambiguous"错误。
要解决这个问题,你需要使用逐元素的比较运算符来比较Series对象中的每个元素。在这种情况下,你可以使用Series对象的.max()方法来找到iaqi1和iaqi2中的最大值。
请修改以下代码:
```python
aqi = max(iaqi1, iaqi2)
```
为:
```python
aqi = pd.Series([iaqi1, iaqi2]).max()
```
这样就可以避免出现"The truth value of a Series is ambiguous"错误,并找到iaqi1和iaqi2中的最大值作为aqi。请确保在使用该代码之前导入pandas库(import pandas as pd)以使用pd.Series()方法。
import sys import re import jieba import codecs import gensim import numpy as np import pandas as pd def segment(doc: str): stop_words = pd.read_csv('data/stopwords.txt', index_col=False, quoting=3, names=['stopword'], sep='\n', encoding='utf-8') stop_words = list(stop_words.stopword) reg_html = re.compile(r'<[^>]+>', re.S) # 去掉html标签数字等 doc = reg_html.sub('', doc) doc = re.sub('[0-9]', '', doc) doc = re.sub('\s', '', doc) word_list = list(jieba.cut(doc)) out_str = '' for word in word_list: if word not in stop_words: out_str += word out_str += ' ' segments = out_str.split(sep=' ') return segments def doc2vec(file_name, model, doc_id): start_alpha = 0.01 infer_epoch = 1000 doc = segment(codecs.open(file_name, 'r', 'utf-8').read()) return model.infer_vector(doc, alpha=start_alpha, steps=infer_epoch) # 计算两个向量余弦值 def similarity(a_vect, b_vect): dot_val = 0.0 a_norm = 0.0 b_norm = 0.0 cos = None for a, b in zip(a_vect, b_vect): dot_val += a * b a_norm += a ** 2 b_norm += b ** 2 if a_norm == 0.0 or b_norm == 0.0: cos = -1 else: cos = dot_val / ((a_norm * b_norm) ** 0.5) return cos def test_model(file1, file2): print('导入模型') model_path = 'tmp/zhwk_news.doc2vec' model = gensim.models.Doc2Vec.load(model_path) vect1 = doc2vec(file1, model, doc_id=0) # 转成句子向量 vect2 = doc2vec(file2, model, doc_id=1) print(vect1.nbytes) # 查看向量大小 print(vect2.nbytes) cos = similarity(vect1, vect2) print('相似度:%0.2f%%' % (cos * 100)) if __name__ == '__main__': file1 = 'data/corpus_test/t1.txt' file2 = 'data/corpus_test/t2.txt' test_model(file1, file2) 报错AttributeError: 'Doc2Vec' object has no attribute 'dv'怎么解决
这个错误可能是因为gensim版本的问题导致的,可以尝试将gensim版本降到3.8.1及以下。具体操作可以使用以下命令:
```
!pip install gensim==3.8.1
```
或者可以在代码中加入以下语句:
```
model.delete_temporary_training_data(keep_doctags_vectors=True, keep_inference=True)
```
这句话可以清除模型中的临时训练数据,可能可以解决这个错误。
阅读全文
相关推荐
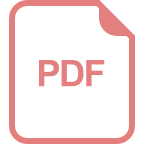
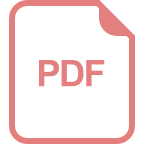
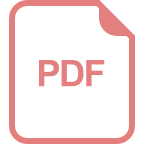










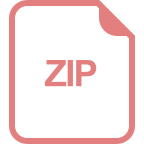