int main(); { int height, weight;,为什么报错缺少函数标题
时间: 2024-04-29 19:23:15 浏览: 28
因为函数定义的语法是以函数名开头,后跟括号和可选的参数列表,再后是函数体。在本例中,函数名称为main,但是在函数名称和左括号之间存在一个分号,这是不正确的语法。正确的语法应该是:
```
int main() {
int height, weight;
// 函数体代码
return 0;
}
```
注意,函数体的语句应该在大括号内。
相关问题
#include <iostream> #include <string.h> #include <stdio.h> using namespace std; class Person { public: Person(char *vn, int vage, float vh, float vw); Person(const Person &vref); void Print(); ~Person(); private: char *pName;//姓名 int age;//年龄 float height;//身高 float weight;//体重 }; //构造函数 Person::Person(char *vn, int vage, float vh, float vw) { age = vage; height = vh; weight = vw; strcpy(pName, vn); cout << age << endl; cout << height << endl; cout << weight << endl; cout << pName << endl; } //拷贝构造函数 Person::Person(const Person &vref) { int len = strlen(vref.pName); pName = new char[len]; age = vref.age; height = vref.height; weight = vref.weight; strcpy(pName, vref.pName); } //输出函数 void Person::Print() { cout << age << endl; cout << height << endl; cout << weight << endl; cout << pName << endl; } Person::~Person() { if (!pName == NULL) { delete[]pName; pName = NULL; } } int main() { string A; double B, C, D; cin >> A >> B >> C >> D; Person A1(A, B, C, D); }
这段代码有一些问题:
1. 在构造函数中,没有为 pName 分配内存,只是复制了指针,这可能导致程序崩溃。
2. 在拷贝构造函数中,同样没有为 pName 分配内存,只是复制了指针,这也可能导致程序崩溃。
3. 在析构函数中,应该检查指针是否为空,如果不为空,才进行释放操作。
4. 在 main 函数中,使用了一个 string 类型的变量 A,但是在 Person 类的构造函数中,要求传入的是 char* 类型的指针,这会导致编译错误。
5. 在 main 函数中,使用了一个 double 类型的变量 B,但是在 Person 类的构造函数中,要求传入的是 int 类型的年龄,这也会导致编译错误。
6. 在 main 函数中,没有调用 Person 类的输出函数,也就是没有输出对象的信息。
下面是修改后的代码:
```c++
#include <iostream>
#include <string.h>
using namespace std;
class Person {
public:
Person(char *vn, int vage, float vh, float vw);
Person(const Person &vref);
void Print();
~Person();
private:
char *pName;//姓名
int age;//年龄
float height;//身高
float weight;//体重
};
//构造函数
Person::Person(char *vn, int vage, float vh, float vw) {
age = vage;
height = vh;
weight = vw;
pName = new char[strlen(vn) + 1];
strcpy(pName, vn);
cout << age << endl;
cout << height << endl;
cout << weight << endl;
cout << pName << endl;
}
//拷贝构造函数
Person::Person(const Person &vref) {
int len = strlen(vref.pName);
pName = new char[len + 1];
age = vref.age;
height = vref.height;
weight = vref.weight;
strcpy(pName, vref.pName);
}
//输出函数
void Person::Print() {
cout << age << endl;
cout << height << endl;
cout << weight << endl;
cout << pName << endl;
}
Person::~Person() {
if (pName != NULL) {
delete[]pName;
pName = NULL;
}
}
int main() {
char A[100];
int B;
float C, D;
cin >> A >> B >> C >> D;
Person A1(A, B, C, D);
A1.Print();
return 0;
}
```
在修改后的代码中,我们为 pName 分配了内存,并在析构函数中进行了释放。同时,我们也将 main 函数中的输入类型和 Person 类的构造函数要求的类型进行了对应。最后,我们调用了 Person 类的输出函数,输出了对象的信息。
#include <iostream> #include <string.h> #include <stdio.h> using namespace std; class Person { public: Person(char *vn, int vage, float vh, float vw); Person(const Person &vref); void Print(); ~Person(); private: char *pName;//姓名 int age;//年龄 float height;//身高 float weight;//体重 }; //构造函数 Person::Person(char *vn, int vage, float vh, float vw) { age = vage; height = vh; weight = vw; strcpy(pName, vn); cout << age << endl; cout << height << endl; cout << weight << endl; cout << pName << endl; } //拷贝构造函数 Person::Person(const Person &vref) { int len = strlen(vref.pName); pName = new char[len]; age = vref.age; height = vref.height; weight = vref.weight; strcpy(pName, vref.pName); } //输出函数 void Person::Print() { cout << age << endl; cout << height << endl; cout << weight << endl; cout << pName << endl; } Person::~Person() { if (!pName == NULL) { delete[]pName; pName = NULL; } } int main() { string A; double B, C, D; cin >> A >> B >> C >> D; Person A1(A, B, C, D); }请指出错误并给出解决办法
在 Person 类的构造函数中,使用了未初始化的指针 pName。应该在构造函数中为 pName 分配内存空间,或者使用字符串对象代替字符指针。
解决办法:
1. 为 pName 分配内存空间
在构造函数中,应该为 pName 分配内存空间,例如:
```
Person::Person(char *vn, int vage, float vh, float vw) {
age = vage;
height = vh;
weight = vw;
pName = new char[strlen(vn)+1]; // 分配内存空间
strcpy(pName, vn);
cout << age << endl;
cout << height << endl;
cout << weight << endl;
cout << pName << endl;
}
```
2. 使用字符串对象代替字符指针
可以使用字符串对象代替字符指针,例如:
```
class Person {
public:
Person(string vn, int vage, float vh, float vw);
Person(const Person &vref);
void Print();
~Person();
private:
string pName; // 姓名
int age; // 年龄
float height; // 身高
float weight; // 体重
};
Person::Person(string vn, int vage, float vh, float vw) {
age = vage;
height = vh;
weight = vw;
pName = vn; // 使用字符串对象代替字符指针
cout << age << endl;
cout << height << endl;
cout << weight << endl;
cout << pName << endl;
}
```
阅读全文
相关推荐
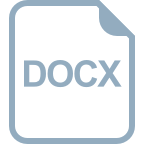
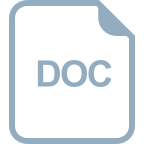
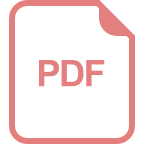














