随机生成一个m行n列的矩阵,进行初等行变换,直到变为行阶梯形矩阵并输出,计算矩阵的秩,如果矩阵为方阵,计算矩阵的行列式,java语言
时间: 2023-12-12 17:23:31 浏览: 68
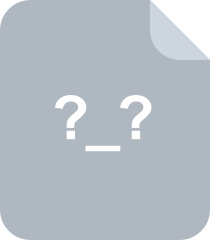
矩阵行列式计算的java实现
好的,以下是Java语言实现随机生成矩阵并进行初等行变换的代码:
```java
import java.util.Random;
public class Matrix {
private double[][] matrix;
private int rows;
private int cols;
public Matrix(int rows, int cols) {
this.rows = rows;
this.cols = cols;
matrix = new double[rows][cols];
Random rand = new Random();
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
matrix[i][j] = rand.nextDouble() * 10;
}
}
}
public void print() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
System.out.print(matrix[i][j] + "\t");
}
System.out.println();
}
}
public void rowReduce() {
int lead = 0;
for (int r = 0; r < rows; r++) {
if (lead >= cols) {
return;
}
int i = r;
while (matrix[i][lead] == 0) {
i++;
if (i == rows) {
i = r;
lead++;
if (lead == cols) {
return;
}
}
}
double[] temp = matrix[r];
matrix[r] = matrix[i];
matrix[i] = temp;
double lv = matrix[r][lead];
for (int j = 0; j < cols; j++) {
matrix[r][j] /= lv;
}
for (int k = 0; k < rows; k++) {
if (k != r) {
double mult = matrix[k][lead];
for (int j = 0; j < cols; j++) {
matrix[k][j] -= mult * matrix[r][j];
}
}
}
lead++;
}
}
public int rank() {
Matrix copy = new Matrix(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
copy.matrix[i][j] = matrix[i][j];
}
}
copy.rowReduce();
int rank = 0;
for (int i = 0; i < rows; i++) {
boolean allZero = true;
for (int j = 0; j < cols; j++) {
if (copy.matrix[i][j] != 0) {
allZero = false;
break;
}
}
if (!allZero) {
rank++;
}
}
return rank;
}
public double determinant() {
if (rows != cols) {
throw new IllegalArgumentException("Matrix must be square");
}
Matrix copy = new Matrix(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
copy.matrix[i][j] = matrix[i][j];
}
}
double det = 1;
for (int i = 0; i < rows; i++) {
if (copy.matrix[i][i] == 0) {
boolean found = false;
for (int j = i + 1; j < rows; j++) {
if (copy.matrix[j][i] != 0) {
found = true;
double[] temp = copy.matrix[i];
copy.matrix[i] = copy.matrix[j];
copy.matrix[j] = temp;
det *= -1;
break;
}
}
if (!found) {
return 0;
}
}
double pivot = copy.matrix[i][i];
det *= pivot;
for (int j = i + 1; j < rows; j++) {
double factor = copy.matrix[j][i] / pivot;
for (int k = i + 1; k < cols; k++) {
copy.matrix[j][k] -= factor * copy.matrix[i][k];
}
}
}
return det;
}
public static void main(String[] args) {
Matrix matrix = new Matrix(3, 4);
System.out.println("Original matrix:");
matrix.print();
matrix.rowReduce();
System.out.println("Row-reduced matrix:");
matrix.print();
System.out.println("Rank: " + matrix.rank());
System.out.println("Determinant: " + matrix.determinant());
}
}
```
这里使用了一个`Matrix`类来表示矩阵,并实现了随机生成矩阵、输出矩阵、进行初等行变换、计算矩阵秩和行列式的功能。在`main`方法中,我们创建了一个3行4列的随机矩阵,并输出了原始矩阵、行阶梯形矩阵、矩阵秩和行列式的值。
阅读全文
相关推荐
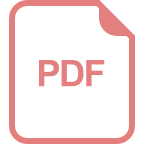







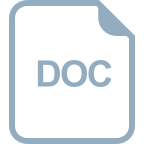
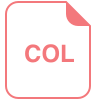

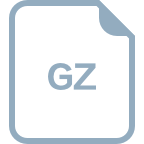
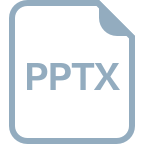
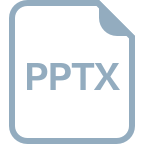
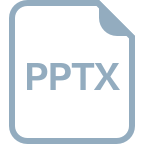
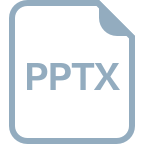
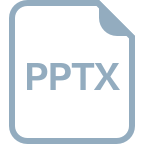