分支限界法解决01背包
时间: 2023-08-27 18:39:23 浏览: 46
好的,针对您的问题,我将为您介绍如何使用分支限界法来解决01背包问题。
01背包问题是指有一个背包,它的容量为C,有n件物品,每件物品的重量为w[i],价值为v[i],将这些物品放入背包中,使得背包中所装物品的总重量不超过C,且总价值最大。
分支限界法是一种搜索算法,它通过对问题空间的搜索,逐步缩小问题的范围,最终得到问题的最优解或近似最优解。使用分支限界法解决01背包问题,首先需要对问题空间进行定义,即定义状态空间树。
状态空间树中的每个节点代表一个状态,每个状态表示某个物品是否放入背包中。如果某个节点代表的状态不满足约束条件,那么这个节点就被剪枝。如果某个节点代表的状态已经达到最终状态,那么这个节点就是叶子节点,可以计算出它的价值,并与当前最优解进行比较。
在计算状态空间树时,每个节点的上界可以通过贪心算法得到。即对于剩余物品中价值最高的物品,如果它的重量小于背包剩余的容量,则将它放入背包中。如果它的重量大于背包剩余的容量,则将它部分放入背包中,使得背包恰好装满。
使用分支限界法解决01背包问题的时间复杂度为O(2^n),其中n为物品的数量。因此,在实际应用中,需要对问题进行剪枝和优化,以减少搜索空间,提高算法效率。
希望这个简单的介绍能对您有所帮助。
相关问题
分支限界法解决01背包 java
分支限界法是一种用于解决组合优化问题的算法,其中包括01背包问题。在Java中实现分支限界法解决01背包问题可以按照以下步骤进行:
1. 定义一个物品类,用于表示每个物品的重量和价值:
```java
class Item {
int weight;
int value;
public Item(int weight, int value) {
this.weight = weight;
this.value = value;
}
}
```
2. 创建一个函数来计算分支限界法解决01背包问题的最大价值:
```java
public static int knapsack(Item[] items, int capacity) {
int n = items.length;
int[][] dp = new int[n + 1][capacity + 1];
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= capacity; j++) {
if (items[i - 1].weight > j) {
dp[i][j] = dp[i - 1][j];
} else {
dp[i][j] = Math.max(dp[i - 1][j], dp[i - 1][j - items[i - 1].weight] + items[i - 1].value);
}
}
}
return dp[n][capacity];
}
```
3. 创建一个测试函数来验证算法的正确性:
```java
public static void main(String[] args) {
Item[] items = new Item[4];
items[0] = new Item(2, 5);
items[1] = new Item(3, 10);
items[2] = new Item(4, 12);
items[3] = new Item(5, 15);
int capacity = 7;
int maxProfit = knapsack(items, capacity);
System.out.println("Maximum profit: " + maxProfit);
}
```
这是一个简单的分支限界法解决01背包问题的Java实现。你可以根据自己的需要进行扩展和修改。希望对你有所帮助!
分支限界法解决01背包问题c
好的,我可以为您介绍一下分支限界法解决01背包问题的思路。
首先,我们需要了解一下01背包问题的定义和特点。01背包问题是指给定一些物品和一个背包,物品有各自的重量和价值,在限定的背包容量下,选择哪些物品能够使得背包中的总价值最大化。这是一个经典的NP完全问题,因此需要采用一些高效的算法来解决。
分支限界法是一种求解组合优化问题的方法,它的基本思想是将问题分解成一系列子问题,每个子问题都是原问题的一个部分,并且这些子问题之间存在某种关系,可以用一棵搜索树来表示。在搜索树的每个节点处,我们记录当前所选物品的重量和价值,以及剩余物品的价值上界。然后,我们根据这些信息来选择下一个节点进行扩展,直到找到最优解或者搜索完整棵树。
具体地,我们可以按照物品的单位价值排序,然后按照贪心策略依次选择物品,直到背包装满或者没有物品可选。在每个节点处,我们计算剩余物品的价值上界,即当前已选择物品的价值加上剩余物品的价值上限,如果这个上限已经小于当前最优解,就可以剪枝,不再扩展该节点。如果这个上限大于当前最优解,则继续扩展该节点,直到找到一个完整的解或者搜索完整棵树。
下面是一个简单的C++代码实现:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
struct Item {
int w, v;
double u;
};
bool cmp(Item a, Item b) {
return a.u > b.u;
}
double maxValue(Item items[], int n, int c) {
sort(items, items + n, cmp);
int curW = 0;
double curV = 0.0;
for (int i = 0; i < n; i++) {
if (curW + items[i].w <= c) {
curW += items[i].w;
curV += items[i].v;
} else {
double remain = c - curW;
curV += remain * items[i].u;
break;
}
}
return curV;
}
double bound(Item items[], int n, int c, int k, int curW, double curV) {
if (curW >= c) {
return 0.0;
}
double bound = curV;
int i;
for (i = k; i < n; i++) {
if (curW + items[i].w <= c) {
bound += items[i].v;
curW += items[i].w;
} else {
double remain = c - curW;
bound += remain * items[i].u;
break;
}
}
if (i < n) {
bound += (c - curW) * items[i].u;
}
return bound;
}
void knapsack(Item items[], int n, int c, double& maxV) {
sort(items, items + n, cmp);
int curW = 0;
double curV = 0.0;
double bestV = 0.0;
int k = 0;
while (true) {
double b = bound(items, n, c, k, curW, curV);
if (b <= bestV) {
break;
}
for (int i = k; i < n; i++) {
if (curW + items[i].w <= c) {
curW += items[i].w;
curV += items[i].v;
}
if (curV > bestV) {
bestV = curV;
}
}
k--;
if (k < 0) {
break;
}
curW -= items[k].w;
curV -= items[k].v;
}
maxV = bestV;
}
int main() {
Item items[4] = {{2, 10}, {3, 5}, {5, 15}, {7, 7}};
int c = 15;
double maxV;
knapsack(items, 4, c, maxV);
cout << "Max value: " << maxV << endl;
return 0;
}
```
这段代码可以求解一个包含4个物品,背包容量为15的01背包问题。其中,Item结构体表示物品的重量w和价值v,以及单位价值u。cmp函数用于按照单位价值从大到小排序,maxValue函数用于计算贪心策略选择物品的最大价值。bound函数用于计算当前节点的价值上界,knapsack函数用于执行分支限界法的主要流程。在这个例子中,最终结果为Max value: 22.5。
希望这个简单的例子能够帮助您更好地理解分支限界法解决01背包问题的思路。
相关推荐
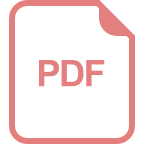
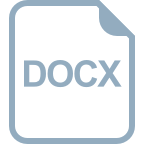











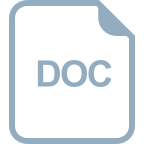