stm32单片机如何通过串口控制LED灯
时间: 2023-09-07 20:11:39 浏览: 312
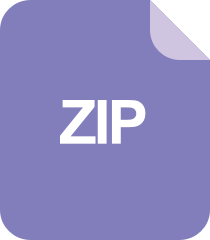
STM32学习——串口控制LED灯.zip

要通过串口控制STM32单片机上的LED灯,需要以下步骤:
1. 首先,需要在STM32单片机上配置串口通信。具体方法如下:
- 配置GPIO引脚为串口通信所需的TX和RX引脚。
- 根据串口通信的参数,配置USART寄存器,包括波特率、数据位、停止位、校验位等。
2. 在主函数中,需要初始化LED引脚为输出模式。
3. 在主函数中,通过串口接收数据,并根据接收到的数据控制LED灯的亮灭。具体方法如下:
- 使用USART_GetFlagStatus函数判断是否接收到了数据。
- 使用USART_ReceiveData函数获取接收到的数据。
- 根据接收到的数据,控制LED灯的亮灭。例如,当接收到字符‘1’时,将LED引脚输出高电平,LED灯亮起;当接收到字符‘0’时,将LED引脚输出低电平,LED灯熄灭。
下面是一个简单的示例代码:
```c
#include "stm32f10x.h"
void USART_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// 使能USART1和GPIOA的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// 配置USART1的TX引脚为复用推挽输出模式,RX引脚为浮空输入模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1的工作参数,包括波特率、数据位、停止位、校验位等
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 使能USART1
USART_Cmd(USART1, ENABLE);
}
void LED_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
// 使能GPIOC的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
// 配置PC13引脚为推挽输出模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_13;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOC, &GPIO_InitStructure);
}
int main(void)
{
USART_Config();
LED_Config();
while (1)
{
// 如果接收到了数据
if (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == SET)
{
// 获取接收到的数据
uint8_t data = USART_ReceiveData(USART1);
// 根据接收到的数据控制LED灯的亮灭
if (data == '1')
GPIO_WriteBit(GPIOC, GPIO_Pin_13, Bit_SET);
else if (data == '0')
GPIO_WriteBit(GPIOC, GPIO_Pin_13, Bit_RESET);
}
}
}
```
在上述代码中,使用USART1配置了串口通信,使用PC13引脚控制了一个LED灯。当接收到字符‘1’时,LED灯亮起;当接收到字符‘0’时,LED灯熄灭。
阅读全文
相关推荐
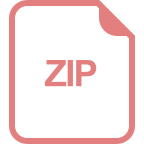
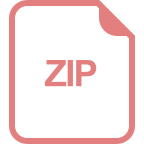















