用Java实现可以重用搜索结果的权值迭代的允许次优解的Anytime repair Astar算法
时间: 2023-06-27 11:08:16 浏览: 51
任意修复A*(Anytime Repair A*,简称ARA*)是一种启发式搜索算法,可以在有限时间内找到最短路径的近似解。它使用了一种称为“任意修复”的技术,可以在搜索过程中重用之前搜索的结果,从而加速搜索过程。
以下是Java实现的ARA*算法,其中包括了允许次优解的权值迭代。
```java
import java.util.*;
public class ARAStar {
private final int[][] graph;
private final int rows, cols;
private final int startRow, startCol, endRow, endCol;
private final double weight;
private final double heuristicWeight;
private final double epsilon;
private double currentEpsilon;
private final PriorityQueue<Node> openList;
private final Map<Node, Double> gScores;
private final Map<Node, Double> fScores;
private final Map<Node, Node> cameFrom;
public ARAStar(int[][] graph, int startRow, int startCol, int endRow, int endCol, double weight, double heuristicWeight, double epsilon) {
this.graph = graph;
this.rows = graph.length;
this.cols = graph[0].length;
this.startRow = startRow;
this.startCol = startCol;
this.endRow = endRow;
this.endCol = endCol;
this.weight = weight;
this.heuristicWeight = heuristicWeight;
this.epsilon = epsilon;
this.currentEpsilon = epsilon;
Comparator<Node> comparator = Comparator.comparingDouble((Node node) -> fScores.get(node));
openList = new PriorityQueue<>(comparator);
gScores = new HashMap<>();
fScores = new HashMap<>();
cameFrom = new HashMap<>();
Node startNode = new Node(startRow, startCol);
gScores.put(startNode, 0.0);
fScores.put(startNode, currentEpsilon * heuristic(startNode));
openList.add(startNode);
}
public List<Node> search() {
while (!openList.isEmpty()) {
Node current = openList.poll();
if (current.row == endRow && current.col == endCol) {
return reconstructPath(current);
}
if (fScores.get(current) > currentEpsilon * gScores.getOrDefault(current, Double.MAX_VALUE)) {
openList.add(current);
continue;
}
for (Node neighbor : getNeighbors(current)) {
double tentativeGScore = gScores.get(current) + getCost(current, neighbor);
if (tentativeGScore < gScores.getOrDefault(neighbor, Double.MAX_VALUE)) {
cameFrom.put(neighbor, current);
gScores.put(neighbor, tentativeGScore);
fScores.put(neighbor, currentEpsilon * tentativeGScore + heuristicWeight * heuristic(neighbor));
if (!openList.contains(neighbor)) {
openList.add(neighbor);
}
}
}
}
return null;
}
private List<Node> reconstructPath(Node current) {
List<Node> path = new ArrayList<>();
path.add(current);
while (cameFrom.containsKey(current)) {
current = cameFrom.get(current);
path.add(0, current);
}
return path;
}
private double getCost(Node node1, Node node2) {
return weight * graph[node2.row][node2.col];
}
private double heuristic(Node node) {
return Math.sqrt(Math.pow(node.row - endRow, 2) + Math.pow(node.col - endCol, 2));
}
private List<Node> getNeighbors(Node node) {
List<Node> neighbors = new ArrayList<>();
if (node.row > 0) {
neighbors.add(new Node(node.row - 1, node.col));
}
if (node.row < rows - 1) {
neighbors.add(new Node(node.row + 1, node.col));
}
if (node.col > 0) {
neighbors.add(new Node(node.row, node.col - 1));
}
if (node.col < cols - 1) {
neighbors.add(new Node(node.row, node.col + 1));
}
return neighbors;
}
public List<Node> findPath() {
List<Node> path = null;
while (path == null) {
path = search();
currentEpsilon += epsilon;
}
return path;
}
static class Node {
final int row, col;
public Node(int row, int col) {
this.row = row;
this.col = col;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Node node = (Node) o;
return row == node.row && col == node.col;
}
@Override
public int hashCode() {
return Objects.hash(row, col);
}
}
}
```
在这个实现中,我们将节点表示为一个简单的包含行和列坐标的类。我们使用了一个优先队列来存储开放列表,其中节点按照f值排序。我们还使用了三个Map来存储g值、f值和每个节点的祖先节点。
在ARA*算法中,我们每次增加ε的值,直到找到一条路径或者搜索完成。我们使用currentEpsilon来存储当前的ε值,并在每次搜索之前将其设置为epsilon。重用之前搜索的结果的技术通过在fScores中存储当前ε值的乘积来实现。
在搜索过程中,我们首先从openList中取出f值最小的节点。如果其f值大于当前ε值下节点的g值和h值之和,则我们将其放回openList中,并继续搜索下一个节点。否则,我们将其标记为已访问,并检查其所有邻居节点。如果某个邻居节点的g值可以通过当前节点获得更小的值,则我们将其更新为新的g值和f值,并将其添加到openList中。
一旦我们找到了终点,我们可以通过追溯每个节点的祖先节点来重建路径。
这就是Java实现的ARA*算法。希望这可以帮助你理解如何在Java中实现这种算法。
相关推荐
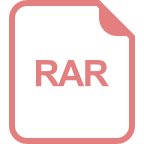
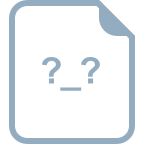














