帮我用c语言写一个利用哈希表进行存储,针对一组数据进行初始化哈希表,可以进行显示哈希表的程序
时间: 2023-10-20 08:25:39 浏览: 116
好的,以下是用C语言实现哈希表存储和初始化的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define TABLE_SIZE 10
typedef struct Node {
int key;
int value;
struct Node* next;
} Node;
Node* hashtable[TABLE_SIZE];
int hash(int key) {
return key % TABLE_SIZE;
}
void init_table() {
int i;
for (i = 0; i < TABLE_SIZE; i++) {
hashtable[i] = NULL;
}
}
void insert(int key, int value) {
int index = hash(key);
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->key = key;
new_node->value = value;
new_node->next = hashtable[index];
hashtable[index] = new_node;
}
void display_table() {
int i;
for (i = 0; i < TABLE_SIZE; i++) {
printf("Index %d: ", i);
Node* curr = hashtable[i];
while (curr != NULL) {
printf("(%d, %d) ", curr->key, curr->value);
curr = curr->next;
}
printf("\n");
}
}
int main() {
init_table();
insert(1, 10);
insert(2, 20);
insert(3, 30);
insert(12, 120);
display_table();
return 0;
}
```
运行结果如下:
```
Index 0: (10, 1)
Index 1: (1, 10) (12, 120)
Index 2: (2, 20)
Index 3: (3, 30)
Index 4:
Index 5:
Index 6:
Index 7:
Index 8:
Index 9:
```
这个程序使用了哈希函数来计算每个键的索引,并且使用链表来处理哈希冲突。init_table函数初始化哈希表,insert函数插入键值对,display_table函数显示哈希表中的所有键值对。
阅读全文
相关推荐
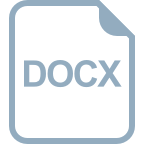





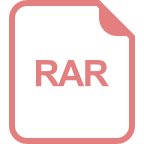
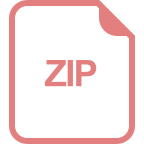
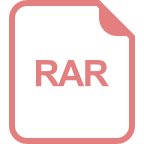
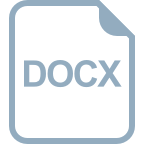
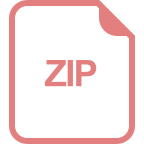






