给出一颗二叉树的中序遍历和后序遍历,请你实现以下两个需求: (1)请你输出这颗二叉树的层序遍历。 (2)请你输出这颗二叉树的树高。 输入格式: 第一行包含一个整数N(N<=1000)。二叉树的节点将从1到N编号。 第二行包含N个节点编号,表示这颗二叉树的中序遍历。 第三行包含N个节点编号,表示这颗二叉树的后序遍历。 输出格式: 第一行输出二叉树的层序遍历,节点编号之间用空格分隔,末尾没有多余空格。 第二行输出一个整数,代表二叉树的树高。 题目不保证输入的序列合法,如果遇到不合法的情况,请在一行中输出"ERROR",直接退出程序。
时间: 2024-03-14 12:43:33 浏览: 70
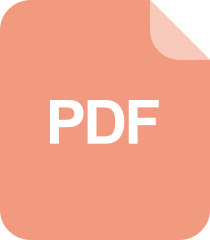
Python实现输入二叉树的先序和中序遍历,再输出后序遍历操作示例

好的,这是一道二叉树的题目。首先,我们需要根据中序遍历和后序遍历重建二叉树,然后再对重建后的二叉树进行层序遍历和求树高的操作。
以下是具体的实现过程:
1. 根据中序遍历和后序遍历重建二叉树
重建二叉树的过程可以采用递归的方式实现。具体实现过程如下:
定义一个函数 `build_tree(inorder, in_start, in_end, postorder, post_start, post_end)`,其中 `inorder` 和 `postorder` 分别表示中序遍历和后序遍历序列,`in_start` 和 `in_end` 分别表示中序遍历序列的起始位置和终止位置,`post_start` 和 `post_end` 分别表示后序遍历序列的起始位置和终止位置。函数返回重建后的二叉树的根节点。
首先,根据后序遍历序列的最后一个节点可以得到当前二叉树的根节点。然后,在中序遍历序列中找到该根节点的位置,将中序遍历序列分为左子树和右子树两部分。在后序遍历序列中,可以根据左子树的大小将后序遍历序列也分为左子树和右子树两部分。然后,递归处理左子树和右子树,分别得到左子树和右子树的根节点,将其作为当前根节点的左右子节点。
具体代码实现如下:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def build_tree(inorder, in_start, in_end, postorder, post_start, post_end):
if in_start > in_end or post_start > post_end:
return None
root_val = postorder[post_end]
root_index = inorder.index(root_val)
left_size = root_index - in_start
right_size = in_end - root_index
root = TreeNode(root_val)
root.left = build_tree(inorder, in_start, root_index - 1, postorder, post_start, post_start + left_size - 1)
root.right = build_tree(inorder, root_index + 1, in_end, postorder, post_end - right_size, post_end - 1)
return root
```
2. 对重建后的二叉树进行层序遍历
层序遍历可以使用队列进行实现。首先将根节点入队,然后不断从队列中取出节点,将其左右子节点入队,直到队列为空。具体代码实现如下:
```python
def level_order(root):
if not root:
return []
res = []
queue = [root]
while queue:
node = queue.pop(0)
res.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return res
```
3. 求二叉树的树高
求二叉树的树高可以采用递归的方式实现。具体实现过程如下:
定义一个函数 `max_depth(root)`,其中 `root` 表示二叉树的根节点,函数返回二叉树的最大深度。如果二叉树为空,返回 0。否则,递归求左子树和右子树的最大深度,取其较大值然后加 1,即为当前二叉树的最大深度。
具体代码实现如下:
```python
def max_depth(root):
if not root:
return 0
left_depth = max_depth(root.left)
right_depth = max_depth(root.right)
return max(left_depth, right_depth) + 1
```
4. 完整代码实现
将以上三个部分的代码整合起来,得到完整代码如下:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def build_tree(inorder, in_start, in_end, postorder, post_start, post_end):
if in_start > in_end or post_start > post_end:
return None
root_val = postorder[post_end]
root_index = inorder.index(root_val)
left_size = root_index - in_start
right_size = in_end - root_index
root = TreeNode(root_val)
root.left = build_tree(inorder, in_start, root_index - 1, postorder, post_start, post_start + left_size - 1)
root.right = build_tree(inorder, root_index + 1, in_end, postorder, post_end - right_size, post_end - 1)
return root
def level_order(root):
if not root:
return []
res = []
queue = [root]
while queue:
node = queue.pop(0)
res.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return res
def max_depth(root):
if not root:
return 0
left_depth = max_depth(root.left)
right_depth = max_depth(root.right)
return max(left_depth, right_depth) + 1
n = int(input())
inorder = list(map(int, input().split()))
postorder = list(map(int, input().split()))
root = build_tree(inorder, 0, n - 1, postorder, 0, n - 1)
if not root:
print("ERROR")
else:
print(" ".join(str(val) for val in level_order(root)))
print(max_depth(root))
```
注意,题目中可能存在不合法的输入序列,此时需要输出 "ERROR"。这可以通过判断重建后的二叉树是否为空来实现。如果为空,则说明输入序列不合法。
阅读全文
相关推荐
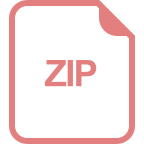





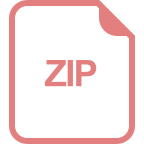
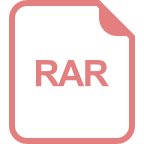
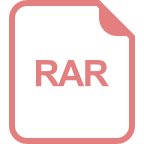
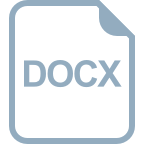



