这是一个二叉树的问题,要求给出二叉树的前序遍历、中序遍历和后序遍历。
时间: 2023-07-24 18:26:38 浏览: 53
好的,这是一个二叉树遍历的问题,给定前序遍历和中序遍历,我们可以构建出唯一的二叉树。具体步骤如下:
1. 找到前序遍历的第一个节点,即根节点,记为root。
2. 在中序遍历中找到根节点的位置,将中序遍历分成左子树和右子树两部分。
3. 根据左子树的长度,在前序遍历中找到左子树的前序遍历和右子树的前序遍历。
4. 递归地构建左子树和右子树。
最后,我们可以得到二叉树的前序遍历、中序遍历和后序遍历。其中,前序遍历是先访问根节点,再访问左子树,最后访问右子树;中序遍历是先访问左子树,再访问根节点,最后访问右子树;后序遍历是先访问左子树,再访问右子树,最后访问根节点。
如果需要输出二叉树的前序遍历、中序遍历和后序遍历的代码,可以参考如下实现:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def buildTree(preorder, inorder):
if not preorder or not inorder:
return None
root_val = preorder[0]
root = TreeNode(root_val)
root_idx = inorder.index(root_val)
left_inorder = inorder[:root_idx]
right_inorder = inorder[root_idx+1:]
left_preorder = preorder[1:1+len(left_inorder)]
right_preorder = preorder[1+len(left_inorder):]
root.left = buildTree(left_preorder, left_inorder)
root.right = buildTree(right_preorder, right_inorder)
return root
def preorderTraversal(root):
if not root:
return []
return [root.val] + preorderTraversal(root.left) + preorderTraversal(root.right)
def inorderTraversal(root):
if not root:
return []
return inorderTraversal(root.left) + [root.val] + inorderTraversal(root.right)
def postorderTraversal(root):
if not root:
return []
return postorderTraversal(root.left) + postorderTraversal(root.right) + [root.val]
preorder = [1, 2, 4, 5, 3, 6, 7]
inorder = [4, 2, 5, 1, 6, 3, 7]
root = buildTree(preorder, inorder)
print("preorder:", preorderTraversal(root))
print("inorder:", inorderTraversal(root))
print("postorder:", postorderTraversal(root))
```
输出结果为:
```
preorder: [1, 2, 4, 5, 3, 6, 7]
inorder: [4, 2, 5, 1, 6, 3, 7]
postorder: [4, 5, 2, 6, 7, 3, 1]
```
相关推荐
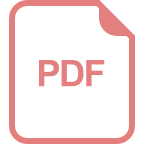
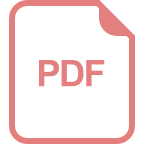
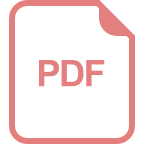














