用C++实现在二维坐标系下一个四边形包含某个点
时间: 2024-05-15 08:19:57 浏览: 77
好的,关于你的问题,可以使用线性代数的方法来解决。首先,我们需要知道四边形的四个顶点坐标,然后使用向量叉积的方法判断该点是否在四边形内部。具体实现可以参考以下代码:
```c
#include <stdio.h>
typedef struct {
double x;
double y;
} Point;
typedef struct {
Point vertices[4];
} Quadrilateral;
double cross_product(Point a, Point b) {
return a.x * b.y - b.x * a.y;
}
int contains_point(Quadrilateral q, Point p) {
double cp1 = cross_product((Point){q.vertices[1].x - q.vertices[0].x, q.vertices[1].y - q.vertices[0].y}, (Point){p.x - q.vertices[0].x, p.y - q.vertices[0].y});
double cp2 = cross_product((Point){q.vertices[2].x - q.vertices[1].x, q.vertices[2].y - q.vertices[1].y}, (Point){p.x - q.vertices[1].x, p.y - q.vertices[1].y});
double cp3 = cross_product((Point){q.vertices[3].x - q.vertices[2].x, q.vertices[3].y - q.vertices[2].y}, (Point){p.x - q.vertices[2].x, p.y - q.vertices[2].y});
double cp4 = cross_product((Point){q.vertices[0].x - q.vertices[3].x, q.vertices[0].y - q.vertices[3].y}, (Point){p.x - q.vertices[3].x, p.y - q.vertices[3].y});
if ((cp1 >= 0 && cp2 >= 0 && cp3 >= 0 && cp4 >= 0) || (cp1 <= 0 && cp2 <= 0 && cp3 <= 0 && cp4 <= 0)) {
return 1;
}
return 0;
}
int main() {
Quadrilateral q = {{{0, 0}, {0, 1}, {1, 1}, {1, 0}}};
Point p = {0.5, 0.5};
if (contains_point(q, p)) {
printf("The point is inside the quadrilateral.\n");
} else {
printf("The point is outside the quadrilateral.\n");
}
return 0;
}
```
当然,以上代码仅供参考,实际应用中还需要考虑各种边界情况和精度问题。
阅读全文
相关推荐
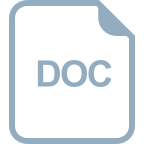
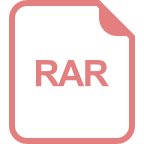
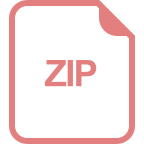
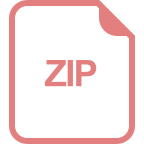
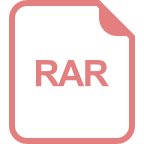
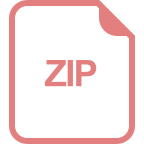
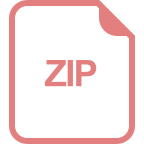
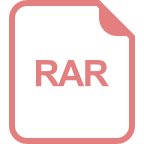
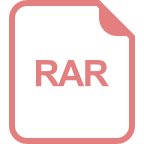
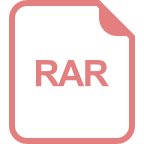
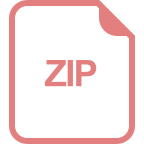
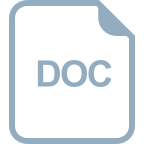
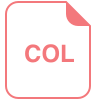
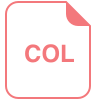
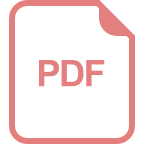