写一个H264的RTP完整代码
时间: 2023-03-12 07:35:57 浏览: 349
答案:H264 RTP完整代码如下:#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <string.h>
#include <fcntl.h>
#include <netdb.h>
#include <arpa/inet.h>
#include <sys/types.h>
#include <inttypes.h>#define MAX_BUF_SIZE 1024//帧类型定义
#define NALU_TYPE_SLICE 1
#define NALU_TYPE_DPA 2
#define NALU_TYPE_DPB 3
#define NALU_TYPE_DPC 4
#define NALU_TYPE_IDR 5
#define NALU_TYPE_SEI 6
#define NALU_TYPE_SPS 7
#define NALU_TYPE_PPS 8
#define NALU_TYPE_AUD 9
#define NALU_TYPE_EOSEQ 10
#define NALU_TYPE_EOSTREAM 11
#define NALU_TYPE_FILL 12//RTP头部
typedef struct
{
//byte 0
unsigned char csrc_len:4; //CSRC的长度
unsigned char extension:1; //是否有扩展
unsigned char padding:1; //是否有填充
unsigned char version:2; //版本号 //byte 1
unsigned char payload:7; //负载类型
unsigned char marker:1; //标记位 //byte 2,3
unsigned short seq_no; //序号 //byte 4-7
unsigned long timestamp; //时间戳 //byte 8-11
unsigned long ssrc; //同步源标识符
} RTP_FIXED_HEADER;//NALU前缀
typedef struct
{
//byte 0
unsigned char TYPE:5; //NALU类型
unsigned char NRI:2; //NALU优先级
unsigned char F:1; //是否有起始前缀} NALU_HEADER;//FU INDICATOR
typedef struct
{
//byte 0
unsigned char TYPE:5; //FU INDICATOR的类型
unsigned char NRI:2; //NALU优先级
unsigned char F:1; //是否有起始前缀} FU_INDICATOR;//FU HEADER
typedef struct
{
//byte 0
unsigned char TYPE:5; //FU HEADER的类型
unsigned char R:1; //是否有起始前缀
unsigned char E:1; //是否有结束前缀
unsigned char S:1; //是否为第一个分片} FU_HEADER;//RTP发送函数
void send_rtp_packet(int sock,unsigned char *buf,int len,unsigned long timestamp)
{
int head_len = 12; //固定头部长度
int send_len; //头部部分
unsigned char rtp_head[head_len];
RTP_FIXED_HEADER *rtp_hdr;
rtp_hdr = (RTP_FIXED_HEADER *)rtp_head; //RTP固定头部
rtp_hdr->version = 2;
rtp_hdr->payload = 96; //发送H264数据,其值为96
rtp_hdr->seq_no = htons(1); //序列号,每发送一个RTP包增1
rtp_hdr->timestamp = htonl(timestamp); //时间戳,每发送一个RTP包更新
rtp_hdr->ssrc = htonl(1); //源标识符,可以自定义 //NALU前缀
NALU_HEADER *nalu_hdr;
nalu_hdr = (NALU_HEADER *)(buf); //FU INDICATOR
FU_INDICATOR *fu_ind;
fu_ind = (FU_INDICATOR *)(buf); //FU HEADER
FU_HEADER *fu_hdr;
fu_hdr = (FU_HEADER *)(buf + 1); //发送一个完整NALU
if(nalu_hdr->F == 0)
{
memcpy(rtp_head+head_len,buf,len);
send_len = head_len + len;
send(sock,rtp_head,send_len,0);
} //分片NALU
else if(nalu_hdr->F == 1)
{
//发送FU INDICATOR
rtp_head[head_len] = fu_ind->F | fu_ind->NRI << 5 | fu_ind->TYPE << 5;
send_len = head_len + 1;
send(sock,rtp_head,send_len,0); //发送FU HEADER
rtp_head[head_len] = fu_hdr->S | fu_hdr->E << 1 | fu_hdr->R << 2 | fu_hdr->TYPE << 5;
send_len = head_len + 1;
send(sock,rtp_head,send_len,0); //发送分片NALU
memcpy(rtp_head+head_len,buf+2,len-2);
send_len = head_len + len - 2;
send(sock,rtp_head,send_len,0);
}
}
阅读全文
相关推荐

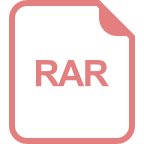
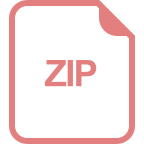
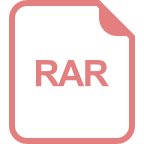
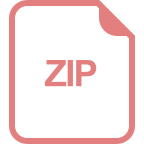
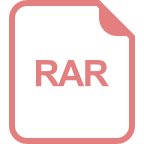
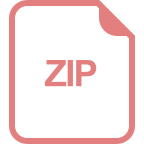
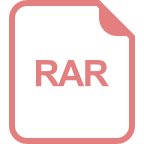
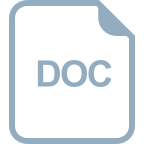





