BMP图像直方图均衡算法(C语言大作业)
时间: 2023-08-31 17:19:46 浏览: 85
好的,我可以为您提供一些关于BMP图像直方图均衡算法的基本思路和C语言实现。
直方图均衡化是一种图像增强技术,它可以使图像的对比度增强,提高图像的质量。直方图均衡化的基本思路是将图像的像素值进行重新分配,使得图像的灰度级分布更加均匀。
下面是一种基于C语言实现的BMP图像直方图均衡算法的伪代码:
1. 读取BMP图像的像素矩阵
2. 统计图像像素矩阵的灰度级直方图
3. 计算直方图均衡化映射表
4. 对原始像素矩阵进行直方图均衡化
5. 将均衡化后的像素矩阵写回BMP图像
下面是一份可以实现直方图均衡化的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#pragma pack(1) //按字节对齐
typedef struct BMPFileHeader {
char bfType[2];
int bfSize;
short bfReserved1;
short bfReserved2;
int bfOffBits;
} BMPFileHeader;
typedef struct BMPInfoHeader {
int biSize;
int biWidth;
int biHeight;
short biPlanes;
short biBitCount;
int biCompression;
int biSizeImage;
int biXPelsPerMeter;
int biYPelsPerMeter;
int biClrUsed;
int biClrImportant;
} BMPInfoHeader;
typedef struct RGB {
unsigned char blue;
unsigned char green;
unsigned char red;
} RGB;
int main()
{
FILE* fp;
BMPFileHeader bmpFileHeader;
BMPInfoHeader bmpInfoHeader;
RGB* image;
RGB* image2;
unsigned char* grayImage;
unsigned char* grayImage2;
int* hist;
int* eqHist;
int* map;
int width, height, size, size2, i, j, k, max;
char filename[100];
// 读取图像文件
printf("Enter the filename of the BMP image: ");
scanf("%s", filename);
fp = fopen(filename, "rb");
if (!fp) {
printf("Error: Failed to open the file.\n");
return -1;
}
// 读取文件头
fread(&bmpFileHeader, sizeof(BMPFileHeader), 1, fp);
if (bmpFileHeader.bfType[0] != 'B' || bmpFileHeader.bfType[1] != 'M') {
printf("Error: Not a BMP file.\n");
return -1;
}
// 读取信息头
fread(&bmpInfoHeader, sizeof(BMPInfoHeader), 1, fp);
if (bmpInfoHeader.biBitCount != 24) {
printf("Error: Not a 24-bit BMP file.\n");
return -1;
}
// 计算图像大小
width = bmpInfoHeader.biWidth;
height = bmpInfoHeader.biHeight;
size = width * height;
size2 = sizeof(unsigned char) * size;
// 读取像素数据
image = (RGB*)malloc(sizeof(RGB) * size);
fread(image, sizeof(RGB), size, fp);
// 将RGB图像转换为灰度图像
grayImage = (unsigned char*)malloc(size2);
for (i = 0; i < size; i++) {
grayImage[i] = (unsigned char)(0.299 * image[i].red + 0.587 * image[i].green + 0.114 * image[i].blue);
}
// 计算灰度级直方图
hist = (int*)malloc(sizeof(int) * 256);
memset(hist, 0, sizeof(int) * 256);
for (i = 0; i < size; i++) {
hist[grayImage[i]]++;
}
// 计算直方图均衡化映射表
eqHist = (int*)malloc(sizeof(int) * 256);
map = (int*)malloc(sizeof(int) * 256);
max = size / 256;
for (i = 0; i < 256; i++) {
eqHist[i] = 0;
for (j = 0; j <= i; j++) {
eqHist[i] += hist[j];
}
map[i] = (int)(eqHist[i] * 255.0 / size + 0.5);
if (eqHist[i] > max) {
eqHist[i] = max;
}
}
// 对灰度图像进行直方图均衡化
grayImage2 = (unsigned char*)malloc(size2);
for (i = 0; i < size; i++) {
grayImage2[i] = (unsigned char)map[grayImage[i]];
}
// 将灰度图像转换为RGB图像
image2 = (RGB*)malloc(sizeof(RGB) * size);
for (i = 0; i < size; i++) {
image2[i].red = grayImage2[i];
image2[i].green = grayImage2[i];
image2[i].blue = grayImage2[i];
}
// 写回图像文件
fseek(fp, sizeof(BMPFileHeader) + sizeof(BMPInfoHeader), SEEK_SET);
fwrite(image2, sizeof(RGB), size, fp);
fclose(fp);
free(image);
free(image2);
free(grayImage);
free(grayImage2);
free(hist);
free(eqHist);
free(map);
printf("Done.\n");
return 0;
}
```
这份代码可以读取一个BMP图像文件,将其转换为灰度图像,进行直方图均衡化,然后将均衡化后的图像写回文件中。注意,这份代码只支持24位的BMP图像文件。
相关推荐
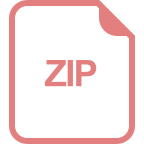














