all_grads = get_or_compute_grads(loss_or_grads, params)报错
时间: 2023-11-17 21:35:50 浏览: 81
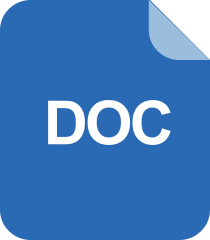
grads 函数
这个报错可能是因为 `loss_or_grads` 或者 `params` 这些参数没有正确的被定义。请确保 `loss_or_grads` 是一个标量张量(scalar tensor)或者梯度列表(gradient list),`params` 是一个变量列表(variable list)。如果传入的参数都正确,请检查它们的类型和形状是否正确。
阅读全文
相关推荐
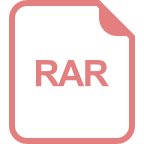



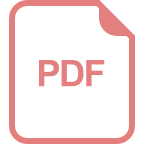











