mean = np.mean(data) std = np.std(data) threshold = 3 * std data = data[abs(data - mean) < threshold]
时间: 2024-06-04 22:12:21 浏览: 11
This code calculates the mean and standard deviation of a dataset (stored in the variable 'data') using the NumPy library. It then sets a threshold value as 3 times the standard deviation. The code next selects only the values in the dataset that are within this threshold range of the mean, effectively removing any outliers. The updated dataset is then stored back into the 'data' variable.
相关问题
mean = np.mean(data) std = np.std(data) threshold = 3 * std data = data[abs(data - mean) < threshold]代码翻译
这段代码的功能是:
1. 计算数据的平均值(mean)和标准差(std)。
2. 计算阈值(threshold),其值为3倍的标准差。
3. 从数据中筛选出绝对值大于等于(abs)平均值与阈值的乘积(mean * threshold)的数据。
具体翻译如下:
```
mean = np.mean(data) # 计算数据的平均值
std = np.std(data) # 计算数据的标准差
threshold = 3 * std # 计算阈值,即3倍的标准差
data = data[abs(data - mean) < threshold] # 从数据中筛选出绝对值小于阈值的数据
```
其中,`np`是numpy库的别名,`mean`、`std`和`data`分别代表数据的平均值、标准差和原始数据。
使用C++ eigen库翻译以下python代码import pandas as pd import numpy as np import time import random def main(): eigen_list = [] data = [[1,2,4,7,6,3],[3,20,1,2,5,4],[2,0,1,5,8,6],[5,3,3,6,3,2],[6,0,5,2,19,3],[5,2,4,9,6,3]] g_csi_corr = np.cov(data, rowvar=True) #print(g_csi_corr) eigenvalue, featurevector = np.linalg.eigh(g_csi_corr) print("eigenvalue:",eigenvalue) eigen_list.append(max(eigenvalue)) #以下代码验证求解csi阈值 eigen_list.append(1.22) eigen_list.append(-54.21) eigen_list.append(8.44) eigen_list.append(-27.83) eigen_list.append(33.12) #eigen_list.append(40.29) print(eigen_list) eigen_a1 = np.array(eigen_list) num1 = len(eigen_list) eigen_a2 = eigen_a1.reshape((-1, num1)) eigen_a3 = np.std(eigen_a2, axis=0) eigen_a4 = eigen_a3.tolist() k = (0.016 - 0.014) / (max(eigen_a4) - min(eigen_a4)) eigen_a5 = [0.014 + k * (i - min(eigen_a4)) for i in eigen_a4] tri_threshold = np.mean(eigen_a5)
#include <iostream>
#include <Eigen/Dense>
using namespace Eigen;
int main()
{
std::vector<double> eigen_list;
MatrixXd data(6, 6);
data << 1, 2, 4, 7, 6, 3,
3, 20, 1, 2, 5, 4,
2, 0, 1, 5, 8, 6,
5, 3, 3, 6, 3, 2,
6, 0, 5, 2, 19, 3,
5, 2, 4, 9, 6, 3;
MatrixXd g_csi_corr = data.transpose() * data / 6.0;
EigenSolver<MatrixXd> es(g_csi_corr);
VectorXd eigenvalue = es.eigenvalues().real();
std::cout << "eigenvalue: " << eigenvalue.transpose() << std::endl;
eigen_list.push_back(eigenvalue.maxCoeff());
eigen_list.push_back(1.22);
eigen_list.push_back(-54.21);
eigen_list.push_back(8.44);
eigen_list.push_back(-27.83);
eigen_list.push_back(33.12);
//eigen_list.push_back(40.29);
std::cout << "eigen_list: ";
for (std::vector<double>::iterator it = eigen_list.begin(); it != eigen_list.end(); ++it)
std::cout << *it << " ";
std::cout << std::endl;
int num1 = eigen_list.size();
MatrixXd eigen_a2 = Map<MatrixXd>(eigen_list.data(), num1, 1);
VectorXd eigen_a3 = eigen_a2.array().rowwise().mean().transpose();
VectorXd eigen_a4 = (eigen_a2 - eigen_a3.replicate(num1, 1)).array().abs().rowwise().mean().transpose();
double k = 0.002 / (eigen_a4.maxCoeff() - eigen_a4.minCoeff());
VectorXd eigen_a5 = 0.014 + k * (eigen_a4.array() - eigen_a4.minCoeff());
double tri_threshold = eigen_a5.mean();
std::cout << "tri_threshold: " << tri_threshold << std::endl;
return 0;
}
相关推荐
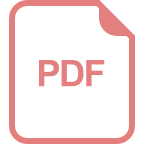
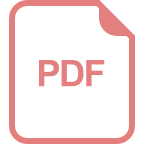













