torch.normal()
时间: 2024-05-09 21:14:30 浏览: 143
`torch.normal()`是PyTorch中的一个函数,用于从正态分布(也称为高斯分布)中采样随机数。它的语法如下:
```
torch.normal(mean, std, out=None)
```
其中,`mean`是正态分布的均值,`std`是正态分布的标准差。可以将`mean`和`std`设置为标量或张量。`out`是可选的输出张量,用于存储采样结果。
例如,以下代码将从均值为0、标准差为1的正态分布中采样一个大小为`(3,2)`的张量:
```
import torch
mean = 0
std = 1
size = (3,2)
x = torch.normal(mean, std, size)
print(x)
```
输出:
```
tensor([[-0.3435, -0.3662],
[-1.3872, 0.0586],
[ 0.5145, -0.9186]])
```
相关问题
torch.normal
torch.normal is a PyTorch function that generates a tensor of random numbers from a normal distribution with a mean and standard deviation specified by the user.
The syntax for torch.normal is as follows:
torch.normal(mean, std, out=None)
- mean: the mean of the normal distribution. This can be a scalar or a tensor with the same shape as the desired output tensor.
- std: the standard deviation of the normal distribution. This can be a scalar or a tensor with the same shape as the desired output tensor.
- out (optional): the output tensor. If provided, the random numbers will be generated into this tensor rather than a new one.
Example usage:
```python
import torch
# Generate a tensor of size (3, 4) with random numbers from a normal distribution
# with mean 0 and standard deviation 1
x = torch.normal(0, 1, size=(3, 4))
print(x)
```
Output:
```
tensor([[-1.0262, -0.7695, 0.1677, -0.0408],
[-1.2485, -1.4555, 0.2709, 0.2705],
[-1.6543, -1.0819, 1.0578, -0.3285]])
```
torch .normal
torch.normal函数可以用于从指定的均值和标准差中生成服从正态分布(高斯分布)的随机数。它的用法如下:
```python
torch.normal(mean, std, size=None, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor
```
参数解释:
- `mean`:一个张量或浮点数,表示正态分布的均值。
- `std`:一个张量或浮点数,表示正态分布的标准差。
- `size`:要生成的随机数的形状。它可以是一个整数或元组。
- `out`:输出张量,用于存储结果。如果未提供,则会创建一个新张量。
- `dtype`:输出张量的数据类型。如果未提供,则使用输入张量的数据类型。
- `layout`:输出张量的布局。
- `device`:输出张量的设备。
- `requires_grad`:指定是否为输出张量开启梯度计算。
示例用法:
```python
import torch
mean = 0
std = 1
size = (3, 4)
random_tensor = torch.normal(mean, std, size=size)
print(random_tensor)
```
这会生成一个形状为(3, 4)的随机张量,其中元素服从均值为0,标准差为1的正态分布。
阅读全文
相关推荐
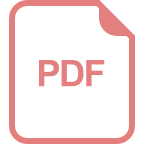
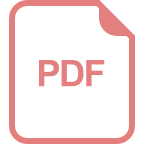
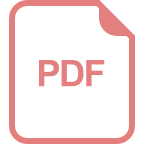













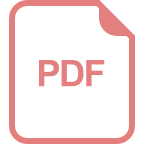