计算1!+2!+3!+4!+….从第100项到第200项之和。java
时间: 2023-09-22 09:06:01 浏览: 31
```java
public class FactorialSum {
public static void main(String[] args) {
long sum = 0;
long factorial = 1;
for (int i = 1; i <= 200; i++) {
factorial *= i;
if (i >= 100) {
sum += factorial;
}
}
System.out.println("The sum of factorials from 100th to 200th is " + sum);
}
}
```
相关问题
用Java语言实现一个线程进行如下运算1+2+3+4+….+200,而另一个线程则每隔一个随机产生的时间(0-100毫秒)读取依次第一个线程的当前运算结果并输出。
以下是用Java语言实现的代码示例:
```java
import java.util.Random;
public class Main {
public static void main(String[] args) {
SumThread sumThread = new SumThread();
PrintThread printThread = new PrintThread(sumThread);
sumThread.start();
printThread.start();
try {
sumThread.join();
printThread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
class SumThread extends Thread {
private int sum;
private Object lock = new Object();
public void run() {
for (int i = 1; i <= 200; i++) {
synchronized (lock) {
sum += i;
}
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public int getSum() {
synchronized (lock) {
return sum;
}
}
}
class PrintThread extends Thread {
private SumThread sumThread;
private Random random = new Random();
public PrintThread(SumThread sumThread) {
this.sumThread = sumThread;
}
public void run() {
while (true) {
try {
Thread.sleep(random.nextInt(100));
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("current sum: " + sumThread.getSum());
}
}
}
```
代码中,`SumThread`代表计算和的线程,`PrintThread`代表输出结果的线程。`SumThread`使用`synchronized`关键字和一个锁来保证在计算和的过程中,任何时候只有一个线程能够修改`sum`的值。`PrintThread`每隔一个随机时间间隔读取`SumThread`的计算结果并输出。
在主函数中,我们启动两个线程并等待它们完成运行。当`SumThread`完成计算后,`join()`函数会等待`PrintThread`完成输出,然后整个程序结束。
写一下springmvc + vue的大文件分片上传代码。要求:1.前端加入MD5;2.用elementui
好的,以下是一个示例代码,使用SpringMVC和Vue实现大文件分片上传,同时前端加入MD5校验,使用ElementUI实现进度条显示。
前端代码:
```vue
<template>
<div>
<el-upload
class="upload-demo"
action="/upload"
:before-upload="beforeUpload"
:on-progress="onProgress"
:on-success="onSuccess"
:file-list="fileList">
<el-button type="primary">点击上传</el-button>
<div slot="tip" class="el-upload__tip">只能上传不超过 200MB 的文件</div>
</el-upload>
<el-progress :percentage="percentage" :stroke-width="30" :text-inside="true"></el-progress>
</div>
</template>
<script>
import SparkMD5 from 'spark-md5'
export default {
data() {
return {
fileList: [],
percentage: 0,
}
},
methods: {
beforeUpload(file) {
return new Promise((resolve, reject) => {
const reader = new FileReader()
const chunkSize = 2 * 1024 * 1024 // 分片大小为2MB
const chunks = Math.ceil(file.size / chunkSize)
const spark = new SparkMD5.ArrayBuffer()
let currentChunk = 0
reader.onload = e => {
spark.append(e.target.result)
currentChunk++
if (currentChunk < chunks) {
loadNext()
} else {
file.md5 = spark.end()
resolve(file)
}
}
reader.onerror = () => {
reject('读取文件出错')
}
function loadNext() {
const start = currentChunk * chunkSize
const end = Math.min(start + chunkSize, file.size)
reader.readAsArrayBuffer(file.slice(start, end))
}
loadNext()
})
},
onProgress(e) {
this.percentage = e.percent
},
onSuccess(response, file) {
this.percentage = 0
this.fileList = []
this.$message.success('上传成功')
},
},
}
</script>
```
在这个示例代码中,我们使用了SparkMD5库来计算文件的MD5,同时在`beforeUpload`方法中,将文件分成多个2MB的分片进行上传,以实现大文件分片上传和MD5校验。在上传过程中,我们使用了ElementUI的进度条组件来显示上传进度。
后端代码:
```java
@Controller
public class UploadController {
private static final String UPLOAD_DIR = "/tmp/uploads/";
private static final int CHUNK_SIZE = 2 * 1024 * 1024;
@RequestMapping(value = "/upload", method = RequestMethod.POST)
@ResponseBody
public ResponseEntity<?> upload(@RequestParam("file") MultipartFile file, @RequestParam("md5") String md5,
@RequestParam(value = "chunk", required = false, defaultValue = "0") int chunk,
@RequestParam(value = "chunks", required = false, defaultValue = "1") int chunks) throws IOException {
String fileName = file.getOriginalFilename();
String filePath = UPLOAD_DIR + fileName;
if (chunk == 0) {
// 如果是第一个分片,检查文件是否存在,如果存在则直接返回
File f = new File(filePath);
if (f.exists()) {
return ResponseEntity.status(HttpStatus.OK).build();
}
} else {
// 如果不是第一个分片,检查文件是否存在,如果不存在则返回错误
File f = new File(filePath);
if (!f.exists()) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("文件不存在");
}
}
if (chunk == 0) {
// 如果是第一个分片,创建文件
File f = new File(filePath);
if (!f.getParentFile().exists()) {
f.getParentFile().mkdirs();
}
f.createNewFile();
}
// 写入分片数据
RandomAccessFile raf = new RandomAccessFile(filePath, "rw");
raf.seek(chunk * CHUNK_SIZE);
raf.write(file.getBytes());
raf.close();
if (chunk == chunks - 1) {
// 如果是最后一个分片,检查文件MD5值是否正确,如果正确则合并文件
String fileMd5 = getFileMd5(filePath);
if (!fileMd5.equals(md5)) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("文件MD5值不正确");
}
mergeFileChunks(filePath, chunks);
}
return ResponseEntity.status(HttpStatus.OK).build();
}
private String getFileMd5(String filePath) throws IOException {
InputStream fis = new FileInputStream(filePath);
byte[] buffer = new byte[1024];
int numRead;
MessageDigest md5;
try {
md5 = MessageDigest.getInstance("MD5");
} catch (NoSuchAlgorithmException e) {
return null;
}
do {
numRead = fis.read(buffer);
if (numRead > 0) {
md5.update(buffer, 0, numRead);
}
} while (numRead != -1);
fis.close();
byte[] md5Bytes = md5.digest();
StringBuilder sb = new StringBuilder();
for (byte b : md5Bytes) {
sb.append(Integer.toString((b & 0xff) + 0x100, 16).substring(1));
}
return sb.toString();
}
private void mergeFileChunks(String filePath, int chunks) throws IOException {
File f = new File(filePath);
FileOutputStream fos = new FileOutputStream(f, true);
byte[] buffer = new byte[1024];
for (int i = 0; i < chunks; i++) {
String chunkFilePath = filePath + "." + i;
File chunk = new File(chunkFilePath);
FileInputStream fis = new FileInputStream(chunk);
int numRead;
while ((numRead = fis.read(buffer)) > 0) {
fos.write(buffer, 0, numRead);
}
fis.close();
chunk.delete();
}
fos.close();
}
}
```
在后端代码中,我们使用了`RandomAccessFile`来实现文件的分片写入和合并,同时在文件合并前使用了`getFileMd5`方法来检查文件的MD5值是否正确。
最后,我们还需要在SpringMVC的配置文件中添加以下配置,以支持大文件上传:
```xml
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="maxUploadSize" value="209715200" />
<property name="maxInMemorySize" value="4096" />
</bean>
```
相关推荐
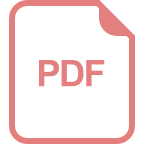
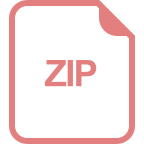
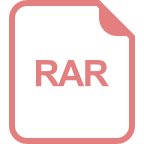
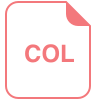

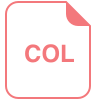
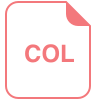
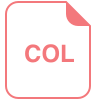








