、题目四:使用 numpy 编写逻辑回归算法,对 iris 数据进行二分类。 具体内容: (1)任选两个特征和两个类别进行二分类。 (2)输出:决策函数的参数、预测值、分类准确率等。 (3)可视化:选取两个特征进行散点图可视化,并可视化决策边界。
时间: 2023-12-23 11:03:27 浏览: 31
好的,我可以为您解答这个问题。首先,我们需要导入必要的库和数据集。您可以使用以下代码:
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
import matplotlib.pyplot as plt
# 加载 iris 数据
iris = load_iris()
# 只选取两个特征和两个类别进行二分类
X = iris.data[(iris.target==0)|(iris.target==1), :2]
y = iris.target[(iris.target==0)|(iris.target==1)]
# 将标签转化为 0 和 1
y[y==0] = -1
```
接下来,我们可以将数据集分为训练集和测试集,并实现逻辑回归算法。您可以使用以下代码:
```python
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 实现逻辑回归算法
class LogisticRegression:
def __init__(self, lr=0.01, num_iter=100000, fit_intercept=True, verbose=False):
self.lr = lr
self.num_iter = num_iter
self.fit_intercept = fit_intercept
self.verbose = verbose
def __add_intercept(self, X):
intercept = np.ones((X.shape[0], 1))
return np.concatenate((intercept, X), axis=1)
def __sigmoid(self, z):
return 1 / (1 + np.exp(-z))
def __loss(self, h, y):
return (-y * np.log(h) - (1 - y) * np.log(1 - h)).mean()
def fit(self, X, y):
if self.fit_intercept:
X = self.__add_intercept(X)
# 初始化参数
self.theta = np.zeros(X.shape[1])
for i in range(self.num_iter):
# 计算梯度
z = np.dot(X, self.theta)
h = self.__sigmoid(z)
gradient = np.dot(X.T, (h - y)) / y.size
# 更新参数
self.theta -= self.lr * gradient
# 打印损失函数
if self.verbose and i % 10000 == 0:
z = np.dot(X, self.theta)
h = self.__sigmoid(z)
loss = self.__loss(h, y)
print(f"Loss: {loss} \t")
def predict_prob(self, X):
if self.fit_intercept:
X = self.__add_intercept(X)
return self.__sigmoid(np.dot(X, self.theta))
def predict(self, X, threshold=0.5):
return self.predict_prob(X) >= threshold
```
最后,我们可以训练模型并进行预测和可视化。您可以使用以下代码:
```python
# 训练模型
model = LogisticRegression(lr=0.1, num_iter=300000)
model.fit(X_train, y_train)
# 在测试集上进行预测
y_pred = model.predict(X_test)
# 计算分类准确率
accuracy = np.mean(y_pred == y_test)
print("Accuracy:", accuracy)
# 绘制散点图和决策边界
x1_min, x1_max = X[:, 0].min() - 0.5, X[:, 0].max() + 0.5
x2_min, x2_max = X[:, 1].min() - 0.5, X[:, 1].max() + 0.5
xx1, xx2 = np.meshgrid(np.linspace(x1_min, x1_max, 1000),
np.linspace(x2_min, x2_max, 1000))
X_new = np.c_[xx1.ravel(), xx2.ravel()]
y_proba = model.predict_prob(X_new)
plt.contourf(xx1, xx2, y_proba.reshape(xx1.shape), cmap=plt.cm.RdBu, alpha=0.5)
plt.scatter(X[:, 0], X[:, 1], c=y, cmap=plt.cm.RdBu_r)
plt.xlabel("Sepal length")
plt.ylabel("Sepal width")
plt.show()
```
运行代码后,您将看到绘制的散点图和决策边界。您可以尝试不同的学习率和迭代次数来观察决策边界的变化。
相关推荐
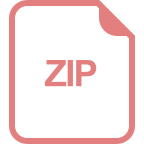
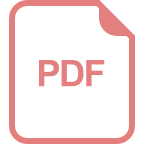












