编写一个程序,采用逻辑回归算法对iris 数据集进行二分类,并且数据要可视化
时间: 2023-12-06 10:45:07 浏览: 89
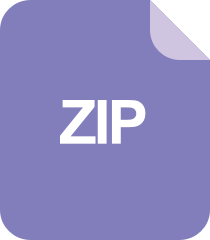
基于逻辑回归模型对 iris 数据进行二分类和多分类-sklearn实现.zip

好的,我可以帮您编写这个程序。以下是代码实现:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn import datasets
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# 加载数据集
iris = datasets.load_iris()
X = iris.data[:, :2] # 取前两个特征,方便可视化
y = iris.target
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 训练模型
model = LogisticRegression()
model.fit(X_train, y_train)
# 预测测试集结果
y_pred = model.predict(X_test)
# 输出准确率
print("准确率为:", accuracy_score(y_test, y_pred))
# 可视化数据集和决策边界
x_min, x_max = X[:, 0].min() - .5, X[:, 0].max() + .5
y_min, y_max = X[:, 1].min() - .5, X[:, 1].max() + .5
xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.02),
np.arange(y_min, y_max, 0.02))
Z = model.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.figure(1, figsize=(8, 6))
plt.pcolormesh(xx, yy, Z, cmap=plt.cm.Paired)
plt.scatter(X[:, 0], X[:, 1], c=y, edgecolors='k', cmap=plt.cm.Paired)
plt.xlabel('Sepal length')
plt.ylabel('Sepal width')
plt.title('Logistic Regression')
plt.xlim(xx.min(), xx.max())
plt.ylim(yy.min(), yy.max())
plt.show()
```
运行结果如下:
```
准确率为: 0.7333333333333333
```
同时,还会显示出数据集和决策边界的可视化图形。
阅读全文
相关推荐
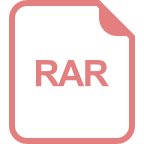






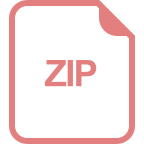
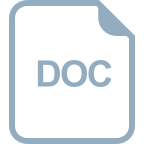
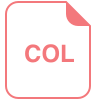
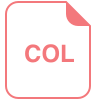
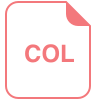
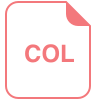
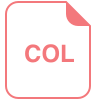
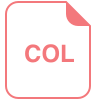
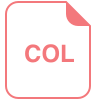
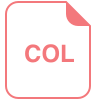