栈应用括号匹配
时间: 2023-07-09 15:13:21 浏览: 100
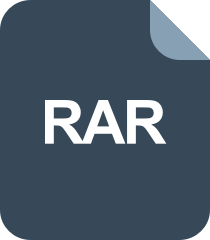
使用顺序栈实现括号匹配

栈可以用来判断括号匹配。具体做法是,遍历字符串,如果遇到左括号就将其入栈,如果遇到右括号就将栈顶元素出栈,并判断其是否与当前右括号匹配。如果栈顶元素与当前右括号不匹配,或者栈已经为空但仍有右括号未匹配,则说明括号不匹配。最后如果栈为空,则说明括号匹配。
以下是一个简单的 C++ 代码示例:
```cpp
#include <iostream>
#include <stack>
#include <string>
using namespace std;
bool isMatch(char left, char right) {
return (left == '(' && right == ')') || (left == '{' && right == '}') || (left == '[' && right == ']');
}
bool checkParentheses(string s) {
stack<char> st;
for (char c : s) {
if (c == '(' || c == '{' || c == '[') {
st.push(c);
} else {
if (st.empty() || !isMatch(st.top(), c)) {
return false;
}
st.pop();
}
}
return st.empty();
}
int main() {
string s = "(({}))";
if (checkParentheses(s)) {
cout << "Parentheses match" << endl;
} else {
cout << "Parentheses do not match" << endl;
}
return 0;
}
```
以上代码中,`isMatch` 函数用于判断左右括号是否匹配,`checkParentheses` 函数用于检查字符串中的括号是否匹配,主函数中对一个括号匹配的字符串进行了测试。
阅读全文
相关推荐
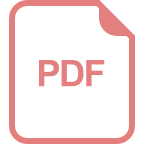
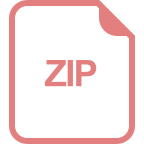















