用python写出读取摄像头视频的代码
时间: 2023-03-13 20:23:02 浏览: 55
使用OpenCV库中的VideoCapture函数可以读取摄像头视频,可以使用以下Python代码: import cv2
# Start capturing the video
cap = cv2.VideoCapture(0)
# Loop runs if capturing has been initialized
while(1):
# Capture the video frame by frame
ret, frame = cap.read()
# Display the resulting frame
cv2.imshow('frame', frame)
# Wait for 'q' to stop the program
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Stop the camera
cap.release()
# Close all windows
cv2.destroyAllWindows()
相关问题
写出python通过opencv调用摄像头实现谷物计数的代码
以下是Python通过OpenCV调用摄像头实现谷物计数的代码示例:
```python
import cv2
camera = cv2.VideoCapture(0) # 初始化摄像头,0代表默认摄像头
while True:
ret, frame = camera.read() # 读取摄像头画面
if not ret:
break
# 处理图像,实现谷物计数
cv2.imshow('Grain Counting', frame) # 显示实时画面
if cv2.waitKey(1) == ord('q'): # 按q键退出
break
camera.release() # 释放摄像头资源
cv2.destroyAllWindows() # 关闭显示窗口
```
希望能帮到您!
用python写一段opencv利用摄像头识别圆环的代码
### 回答1:
下面是一段使用 OpenCV 识别圆环的 Python 代码:
```python
import cv2
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取每一帧
ret, frame = cap.read()
# 转化为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 高斯模糊,平滑图像
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
# Canny边缘检测
edges = cv2.Canny(blurred, 50, 150)
# 在图像中查找圆形
circles = cv2.HoughCircles(edges, cv2.HOUGH_GRADIENT, 1, 20, param1=50, param2=30, minRadius=0, maxRadius=0)
# 如果找到圆形
if circles is not None:
# 将检测到的圆形数量存储在circles中
circles = np.round(circles[0, :]).astype("int")
# 遍历每一个圆形
for (x, y, r) in circles:
# 画出圆形
cv2.circle(frame, (x, y), r, (0, 255, 0), 2)
cv2.rectangle(frame, (x - 5, y - 5), (x + 5, y + 5), (0, 128, 255), -1)
# 显示图像
cv2.imshow("Frame", frame)
# 检测键盘输入,如果是 q 则退出循环
key = cv2.waitKey(1) & 0xFF
if key == ord("q"):
break
# 释放摄像头资源
cap.release()
# 关闭所有窗口
cv2.destroyAllWindows()
```
这段代码实现了以下步骤:
1. 打开摄像头
2. 读取摄像头捕捉到的帧,并将其转换为灰度图像
3. 对图像进行高斯模
### 回答2:
下面是使用Python编写的使用OpenCV识别圆环的代码:
```python
import cv2
import numpy as np
def detect_circle():
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 从摄像头读取一帧图像
ret, frame = cap.read()
if not ret:
break
# 将图像转换为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 对图像进行模糊处理,去除噪声
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
# 使用Hough圆变换检测圆环
circles = cv2.HoughCircles(blurred, cv2.HOUGH_GRADIENT, dp=1, minDist=100, param1=50, param2=30, minRadius=50, maxRadius=200)
# 如果检测到圆环,则画出圆环的轮廓
if circles is not None:
circles = np.round(circles[0, :]).astype("int")
for (x, y, r) in circles:
# 画出圆形轮廓
cv2.circle(frame, (x, y), r, (0, 255, 0), 4)
# 画出圆心
cv2.circle(frame, (x, y), 2, (0, 0, 255), 3)
# 显示图像
cv2.imshow("Circle Detection", frame)
# 按下ESC键退出循环
if cv2.waitKey(1) == 27:
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
if __name__ == '__main__':
detect_circle()
```
使用此代码,打开电脑上的摄像头,检测图像中的圆环,并在屏幕上用绿色的圆线标记出来,用红色的点标记圆心。你可以调整参数来适应不同的情况。
相关推荐
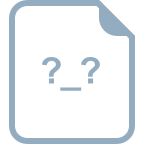
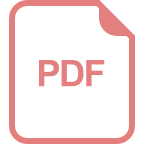










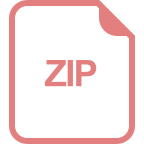