使用c++模拟ATM实现取款、存款、查询帐户余额(当然,ATM还有转帐修改密码等功能) 。3. 课程设计要求: (1)定义最初类: 帐户类AccoutItem:定义4各私有成员:帐户、口令、姓名和存款余额,同时定义公有成员构造函数和对象显示函数Display 及存/取函数。 (2)操作过程: ① 登陆(用户插卡),进行身份验证。 ② 用户对ATM进行取款、存款、查询帐户余额操作。 ③ 退出系统。
时间: 2024-03-20 20:45:30 浏览: 43
好的,以下是一个简单的ATM模拟程序,实现了取款、存款和查询余额功能:
```c++
#include <iostream>
#include <string>
using namespace std;
class AccountItem {
private:
string account;
string password;
string name;
double balance;
public:
AccountItem(string a, string p, string n, double b) {
account = a;
password = p;
name = n;
balance = b;
}
void display() {
cout << "Account: " << account << endl;
cout << "Name: " << name << endl;
cout << "Balance: " << balance << endl;
}
void deposit(double amount) {
balance += amount;
}
void withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
} else {
cout << "Insufficient balance!" << endl;
}
}
bool verify(string a, string p) {
return account == a && password == p;
}
};
int main() {
AccountItem account("123456789", "password", "John", 5000.0);
string input_account, input_password;
cout << "Please insert your card and enter your account and password:" << endl;
cin >> input_account >> input_password;
if (account.verify(input_account, input_password)) {
cout << "Login success!" << endl;
while (true) {
int choice;
cout << "Please choose an operation: 1. Deposit 2. Withdraw 3. Check balance 4. Exit" << endl;
cin >> choice;
if (choice == 1) {
double amount;
cout << "Please enter the deposit amount: ";
cin >> amount;
account.deposit(amount);
cout << "Deposit success!" << endl;
} else if (choice == 2) {
double amount;
cout << "Please enter the withdraw amount: ";
cin >> amount;
account.withdraw(amount);
} else if (choice == 3) {
account.display();
} else if (choice == 4) {
cout << "Thank you for using our ATM!" << endl;
break;
} else {
cout << "Invalid choice, please try again!" << endl;
}
}
} else {
cout << "Login failed, please try again!" << endl;
}
return 0;
}
```
程序中创建了一个AccountItem类,包含了4个私有成员:账户、口令、姓名和存款余额,以及公有成员构造函数、对象显示函数Display和存/取函数。在主函数中,创建了一个AccountItem对象,并通过输入身份验证来进行登录操作。如果登录成功,进入一个循环,等待用户选择操作,包括存款、取款和查询余额。用户可以反复进行操作,直到选择退出程序。
希望这个程序可以帮到你!
相关推荐
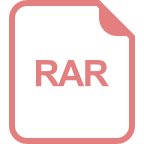
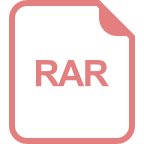
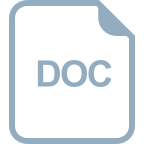















