用c++编写程序实现棋盘密码的加密解密
时间: 2023-08-05 14:21:11 浏览: 143
棋盘密码是一种简单的加密算法,其实现比较简单,以下是用 C++ 实现棋盘密码的加密解密的示例代码:
```c++
#include <iostream>
#include <string>
#include <cmath>
using namespace std;
// 加密函数
string encrypt(string plaintext, string key) {
string ciphertext = "";
int row = sqrt(plaintext.length()) + 1; // 计算棋盘的行数
int col = row; // 棋盘的列数等于行数
int index = 0; // 明文字符索引
bool *used = new bool[row * col]; // 标识格子是否被使用
for (int i = 0; i < row * col; i++) {
used[i] = false;
}
// 生成棋盘
char **board = new char*[row];
for (int i = 0; i < row; i++) {
board[i] = new char[col];
for (int j = 0; j < col; j++) {
if (i == row - 1 && j == col - 1) {
board[i][j] = ' '; // 最后一个格子放空格
} else {
board[i][j] = key[index++];
}
}
}
// 加密明文
for (int i = 0; i < plaintext.length(); i += 2) {
char c1 = plaintext[i];
char c2 = ' ';
if (i + 1 < plaintext.length()) {
c2 = plaintext[i + 1];
}
int r1 = -1, c1 = -1, r2 = -1, c2 = -1;
// 查找字符所在的格子
for (int j = 0; j < row; j++) {
for (int k = 0; k < col; k++) {
if (board[j][k] == c1) {
r1 = j;
c1 = k;
}
if (board[j][k] == c2) {
r2 = j;
c2 = k;
}
}
}
// 将格子的行列号转换为数字串
string s1 = to_string(r1) + to_string(c1);
string s2 = to_string(r2) + to_string(c2);
// 将数字串转换为字符
for (int j = 0; j < s1.length(); j++) {
ciphertext += s1[j];
}
for (int j = 0; j < s2.length(); j++) {
ciphertext += s2[j];
}
}
return ciphertext;
}
// 解密函数
string decrypt(string ciphertext, string key) {
string plaintext = "";
int row = sqrt(ciphertext.length() / 2) + 1; // 计算棋盘的行数
int col = row; // 棋盘的列数等于行数
int index = 0; // 密文字符索引
bool *used = new bool[row * col]; // 标识格子是否被使用
for (int i = 0; i < row * col; i++) {
used[i] = false;
}
// 生成棋盘
char **board = new char*[row];
for (int i = 0; i < row; i++) {
board[i] = new char[col];
for (int j = 0; j < col; j++) {
if (i == row - 1 && j == col - 1) {
board[i][j] = ' '; // 最后一个格子放空格
} else {
board[i][j] = key[index++];
}
}
}
// 解密密文
for (int i = 0; i < ciphertext.length(); i += 4) {
int r1 = ciphertext[i] - '0';
int c1 = ciphertext[i + 1] - '0';
int r2 = ciphertext[i + 2] - '0';
int c2 = ciphertext[i + 3] - '0';
// 查找格子中的字符
char c1 = board[r1][c1];
char c2 = board[r2][c2];
plaintext += c1;
if (c2 != ' ') {
plaintext += c2;
}
}
return plaintext;
}
int main() {
string plaintext = "Hello world!";
string key = "abcdefghijklmnopqrstuvwxyz";
string ciphertext = encrypt(plaintext, key);
cout << "加密后的密文:" << ciphertext << endl;
plaintext = decrypt(ciphertext, key);
cout << "解密后的明文:" << plaintext << endl;
return 0;
}
```
注:上述代码只是示例代码,实际使用时需要考虑字符集、边界条件等问题。
阅读全文
相关推荐
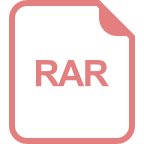




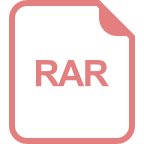
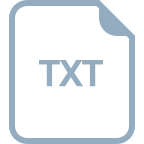
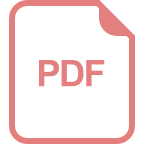
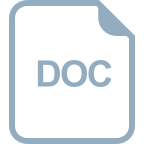
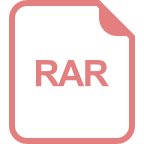
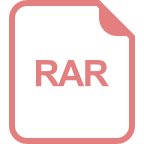
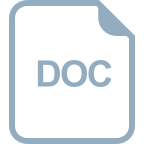
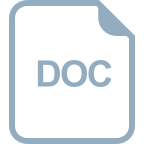