理发师问题的描述:一个理发店接待室有n张椅子,工作室有1张椅子;没有顾客时,理发师睡觉;第一个顾客来到时,必须将理发师唤醒;顾客来时如果还有空座的话,他就坐在一个座位上等待;如果顾客来时没有空座位了,他就离开,不理发了;当理发师处理完所有顾客,而又没有新顾客来时,他又开始睡觉。C语言实现
时间: 2024-02-07 07:04:07 浏览: 154
下面是一个C语言实现的例子,使用了pthread库进行多线程编程和互斥锁控制:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h>
#define N_CHAIRS 5 // 接待室的椅子数量
#define WORKING_CHAIR 1 // 工作室的椅子数量
int n_waiting = 0; // 等待的顾客数
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; // 互斥锁
pthread_cond_t barber_cv = PTHREAD_COND_INITIALIZER; // 理发师条件变量
pthread_cond_t customer_cv = PTHREAD_COND_INITIALIZER; // 顾客条件变量
void *barber(void *arg);
void *customer(void *arg);
int main(int argc, char *argv[]) {
pthread_t barber_thread;
pthread_t customer_threads[10]; // 假设有10个顾客
// 创建理发师线程
pthread_create(&barber_thread, NULL, barber, NULL);
// 创建顾客线程
for (int i = 0; i < 10; i++) {
pthread_create(&customer_threads[i], NULL, customer, (void*)(long)i);
usleep(rand() % 1000); // 随机等待一段时间再创建下一个顾客
}
// 等待所有线程结束
pthread_join(barber_thread, NULL);
for (int i = 0; i < 10; i++) {
pthread_join(customer_threads[i], NULL);
}
return 0;
}
void *barber(void *arg) {
while (1) {
pthread_mutex_lock(&mutex);
if (n_waiting == 0) { // 如果没有顾客,理发师睡觉
printf("Barber is sleeping.\n");
pthread_cond_wait(&barber_cv, &mutex); // 等待顾客唤醒他
}
n_waiting--; // 取出一位等待的顾客
printf("Barber is cutting hair.\n");
pthread_mutex_unlock(&mutex);
usleep(rand() % 1000); // 理发师处理顾客的时间
}
}
void *customer(void *arg) {
int id = (int)(long)arg;
pthread_mutex_lock(&mutex);
if (n_waiting < N_CHAIRS) { // 如果有空座位,就坐下等待
printf("Customer %d is waiting.\n", id);
n_waiting++;
pthread_cond_signal(&barber_cv); // 唤醒理发师
pthread_mutex_unlock(&mutex);
pthread_mutex_lock(&mutex);
while (n_waiting > 0) { // 等待理发师处理
pthread_cond_wait(&customer_cv, &mutex);
}
printf("Customer %d is leaving.\n", id);
pthread_mutex_unlock(&mutex);
} else { // 如果没有空座位,离开
printf("Customer %d is leaving.\n", id);
pthread_mutex_unlock(&mutex);
}
return NULL;
}
```
在这个例子中,我们创建了一个理发师线程和10个顾客线程。理发师线程的实现比较简单,就是不断地循环等待顾客的到来,并处理顾客的请求。顾客线程首先需要获取互斥锁,然后检查是否有空座位。如果有空座位,他就坐下等待,并唤醒理发师。如果没有空座位,他就离开。当理发师处理完一个顾客后,他通过条件变量唤醒等待的顾客,并释放互斥锁。
阅读全文
相关推荐







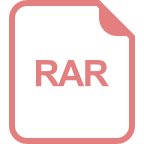




