用C语言实现人机对战乒乓球小游戏
时间: 2023-10-10 12:08:39 浏览: 46
好的,下面是一个简单的用C语言实现的乒乓球小游戏:
```c
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <windows.h>
#include <time.h>
#define WIDTH 40 // 场景宽度
#define HEIGHT 20 // 场景高度
int ball_x; // 球的横坐标
int ball_y; // 球的纵坐标
int ball_vx; // 球的横向速度
int ball_vy; // 球的纵向速度
int paddle1_pos; // 左边球拍的位置
int paddle2_pos; // 右边球拍的位置
int score1; // 左边玩家的得分
int score2; // 右边玩家的得分
void gotoxy(int x, int y) { // 设置光标位置
COORD pos = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void draw_scene() { // 绘制场景
int i, j;
system("cls"); // 清屏
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
if (i == 0 || i == HEIGHT - 1) {
printf("-");
} else if (j == 0 || j == WIDTH - 1) {
printf("|");
} else if (i == ball_y && j == ball_x) {
printf("o");
} else if (j == 2 && i >= paddle1_pos && i < paddle1_pos + 4) {
printf("|");
} else if (j == WIDTH - 3 && i >= paddle2_pos && i < paddle2_pos + 4) {
printf("|");
} else {
printf(" ");
}
}
printf("\n");
}
printf("Score: %d - %d", score1, score2); // 显示得分
}
void init_game() { // 初始化游戏
ball_x = WIDTH / 2;
ball_y = HEIGHT / 2;
ball_vx = 1;
ball_vy = 1;
paddle1_pos = HEIGHT / 2 - 2;
paddle2_pos = HEIGHT / 2 - 2;
score1 = 0;
score2 = 0;
draw_scene();
}
void update_ball() { // 更新球的位置
ball_x += ball_vx;
ball_y += ball_vy;
if (ball_y == 1 || ball_y == HEIGHT - 2) { // 碰到上下墙壁
ball_vy = -ball_vy;
}
if (ball_x == 2) { // 碰到左边球拍
if (ball_y >= paddle1_pos && ball_y < paddle1_pos + 4) {
ball_vx = -ball_vx;
} else {
score2++;
init_game();
}
}
if (ball_x == WIDTH - 3) { // 碰到右边球拍
if (ball_y >= paddle2_pos && ball_y < paddle2_pos + 4) {
ball_vx = -ball_vx;
} else {
score1++;
init_game();
}
}
}
void update_paddle() { // 更新球拍的位置
if (_kbhit()) { // 判断是否有按键按下
char key = _getch();
switch (key) {
case 'w':
if (paddle1_pos > 1) {
paddle1_pos--;
}
break;
case 's':
if (paddle1_pos < HEIGHT - 5) {
paddle1_pos++;
}
break;
case 'i':
if (paddle2_pos > 1) {
paddle2_pos--;
}
break;
case 'k':
if (paddle2_pos < HEIGHT - 5) {
paddle2_pos++;
}
break;
}
}
}
int main() {
srand(time(NULL)); // 设置随机数种子
init_game();
while (1) {
update_ball();
update_paddle();
draw_scene();
Sleep(50); // 暂停50毫秒,防止过快刷新屏幕
}
return 0;
}
```
该程序使用了Windows API中的`SetConsoleCursorPosition`函数和`Sleep`函数,需要在Windows操作系统下编译运行。在游戏中,玩家1使用键盘上的w和s键控制左边的球拍,玩家2使用键盘上的i和k键控制右边的球拍。每当一方未能接到球时,另一方就会得分,并重新开始游戏。
相关推荐
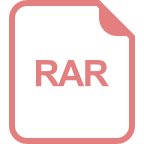














