jacobi迭代法c语言代码
时间: 2023-09-04 18:06:46 浏览: 37
以下是Jacobi迭代法的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_ITERATIONS 1000 //最大迭代次数
#define TOLERANCE 1.0e-6 //容差
#define N 3 //方程组的未知数个数
void Jacobi(double A[][N], double b[], double x[]) {
int i, j, k;
double sum;
double *x0 = (double *)malloc(N * sizeof(double)); //存储上一次迭代的结果
for (k = 0; k < MAX_ITERATIONS; k++) {
for (i = 0; i < N; i++) {
sum = 0.0;
for (j = 0; j < N; j++) {
if (j != i) {
sum += A[i][j] * x0[j];
}
}
x[i] = (b[i] - sum) / A[i][i];
}
//检查迭代是否收敛
double error = 0.0;
for (i = 0; i < N; i++) {
error += fabs(x[i] - x0[i]);
}
if (error < TOLERANCE) {
printf("Jacobi method converged after %d iterations.\n", k + 1);
return;
}
//将本次迭代的结果存储到x0中,作为下一次迭代的初始值
for (i = 0; i < N; i++) {
x0[i] = x[i];
}
}
printf("Jacobi method did not converge after %d iterations.\n", MAX_ITERATIONS);
return;
}
int main() {
double A[N][N] = {{4.0, -1.0, 0.0},
{2.0, 7.0, -2.0},
{0.0, -3.0, 5.0}};
double b[N] = {2.0, -3.0, 7.0};
double x[N] = {0.0};
Jacobi(A, b, x);
printf("The solution is:\n");
for (int i = 0; i < N; i++) {
printf("x[%d] = %f\n", i, x[i]);
}
return 0;
}
```
这里仅提供Jacobi迭代法的基本实现,实际使用中还需要考虑更多的细节,比如矩阵是否对称正定,是否需要设置初始值等问题。
相关推荐
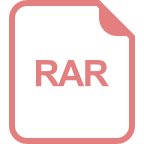











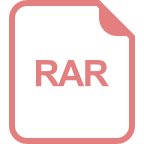