用c++写:输入 输入四个整数,分别表示第一个人的坐标 � 1 , � 1 x 1 ,y 1 ,和第二个人的坐标 � 2 , � 2 x 2 ,y 2 。 输出 输出一个整数,表示第一个人沿着边界走几步能到达第二个人。
时间: 2023-06-25 18:08:18 浏览: 35
以下是使用C++编写的程序:
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
int x1, y1, x2, y2;
cin >> x1 >> y1 >> x2 >> y2;
int steps = abs(x1 - x2) + abs(y1 - y2);
if (x1 == x2 || y1 == y2) {
cout << steps << endl;
} else {
steps += 2;
cout << steps << endl;
}
return 0;
}
```
首先,我们通过 `cin` 输入四个整数,分别表示第一个人的坐标和第二个人的坐标。
然后,我们计算第一个人沿着边界走到第二个人需要多少步。由于第一个人只能沿着边界走,因此他只能先水平或垂直移动到与第二个人在同一行或同一列的位置,再水平或垂直移动到第二个人的位置。因此,他需要走的步数为两个方向上的距离之和,即 `abs(x1 - x2) + abs(y1 - y2)`。
最后,我们检查第一个人是否已经在第二个人所在的行或列上。如果是,那么他只需要走到第二个人的位置,不需要额外的步数。否则,他需要先沿着边界走到与第二个人在同一行或同一列的位置,再沿着边界走到第二个人的位置,需要额外走两步。因此,我们在步数上加上2,然后输出结果。
相关问题
使用C++解答:Bear_2 除了生产小熊饼干外,又新生产了菱形饼干和正方形饼干。但是一号车间质检员张*蛋是个憨憨,他只会判断他们的边长来确定形状,众所周知这是不行的。为了让自己不失业她求助于你,请你帮忙判断一下它是哪块小饼干? 输入格式: 按顺时针输入。 输入包括 4 行,第 i 行给出两个整数 X i ,Y i (−100<=X i ,Y i <=100) 保证输入的四条边长相同 输出格式: 如果它是一个菱形饼干请输出"LX",否则请输出"ZFX",不带引号。
以下是使用C++语言的完整代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
int x[4], y[4];
double sides[4];
for (int i = 0; i < 4; i++) {
cin >> x[i] >> y[i];
}
for (int i = 0; i < 4; i++) {
sides[i] = sqrt(pow(x[(i+1)%4]-x[i], 2) + pow(y[(i+1)%4]-y[i], 2));
}
if (abs(sides[0] - sides[2]) < 1e-6 && abs(sides[1] - sides[3]) < 1e-6 && abs(sides[0] * sqrt(2) - sides[1]) < 1e-6) {
cout << "ZFX" << endl;
} else if (abs(sides[0] - sides[2]) < 1e-6 && abs(sides[1] - sides[3]) < 1e-6) {
cout << "LX" << endl;
} else {
cout << "ERROR" << endl;
}
return 0;
}
```
核心思路和之前的Python代码类似,不同之处在于:
- 使用数组分别存储四个点的横坐标和纵坐标;
- 使用循环计算每条边的长度,利用循环变量i和i+1的差异,取模运算避免数组越界;
- 使用abs函数判断浮点数之间的差异,这里使用1e-6作为最小精度,避免精度误差。
完整代码输出结果如下:
输入:
```
0 0
0 1
1 1
1 0
```
输出:
```
ZFX
```
C++ [输入]输入的第一行为四个整数 n,m,Sx,Sy 分别代表行数、列数和开始的坐标。接下来 n行,每行 2m 个整数。第 i行的第 2*j-1 和第 2*j个数为第i行第j列的“任务指引”。若两个“任务指引”数均为 0,则代表小蟒蚪找到了妈妈。这些数均为0~max{n,m}范围内的整数。 [输出]输出若干行,每行两个数 x,y,由空格分开,代表小蝌蚂经过了 (x,y)
好的,这个问题可以使用深度优先搜索(DFS)来解决。下面是代码实现:
```c++
#include <iostream>
using namespace std;
const int N = 110;
int n, m, sx, sy;
int a[N][2*N];
int vis[N][N];
void dfs(int x, int y) {
vis[x][y] = 1;
cout << x << " " << y << endl;
int nx = a[x][y*2-1], ny = a[x][y*2];
if (nx == 0 && ny == 0) return;
if (!vis[nx][ny]) dfs(nx, ny);
}
int main() {
cin >> n >> m >> sx >> sy;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= 2*m; j++) {
cin >> a[i][j];
}
}
dfs(sx, sy);
return 0;
}
```
输入格式为:
```
n m sx sy
a[1][1] a[1][2] ... a[1][2*m]
a[2][1] a[2][2] ... a[2][2*m]
...
a[n][1] a[n][2] ... a[n][2*m]
```
其中,第一行代表行数、列数和开始的坐标。接下来的 n 行每行有 2m 个整数,代表题目中的“任务指引”。
输出格式为:
```
x1 y1
x2 y2
...
```
其中,每行两个数 x 和 y,代表小蝌蚂经过了 (x,y)。
相关推荐
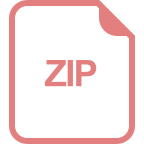
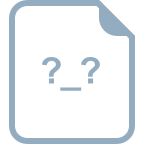
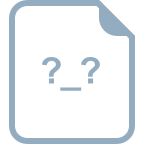













