11.给定两个正整数a,b(1<=a<=b<=10^5),请你数出在a到b之间,十个阿拉伯数字分别出现了多少次。比如,当a=11,b=20时,a和b之间的数有[11,12,13,14,15,16,17,18,19,20],那么0-9这10个数出现的次数分别是1,10,2,1,1,1,1,1,1,1。现在给你a和b,请你输出十个阿拉伯数字分别出现的次数;分十行输出,第一行表示0出现的次数,第二行表示1出现的次数,....,最后一行表示9出现的次数。【输入示例】a=11,b=20【输出示例】1,10,2,1,1,1,1,1,1,1(按行输出)用python编程并解释
时间: 2023-09-18 22:10:24 浏览: 110
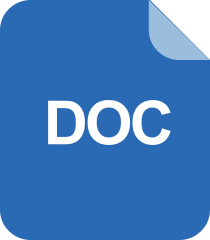
求两个正整数a 和 b的最大公约数。
代码实现如下:
```
a, b = map(int, input().strip().split())
# 初始化每个数字出现的次数为0
count = [0] * 10
for num in range(a, b+1):
# 将数字转化为字符串
num_str = str(num)
# 遍历字符串中的每个字符
for char in num_str:
# 将字符转化为数字,并在列表中加1
count[int(char)] += 1
# 按行输出每个数字出现的次数
for i in range(10):
print(count[i])
```
代码解释如下:
1. 首先读入输入的两个正整数a和b;
2. 初始化一个长度为10的列表count,用于存储每个数字出现的次数;
3. 使用range函数遍历区间[a,b]中的每个数字;
4. 将数字转化为字符串,并遍历字符串中的每个字符;
5. 将字符转化为数字,并将相应的数字在列表count中加1;
6. 最后按照题目要求输出每个数字出现的次数,即按行输出列表count中的每个元素。
阅读全文
相关推荐
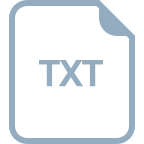
















