二叉树的构建和遍历操作 实验目的:掌握二叉树的构建及遍历操作。 实验内容:按先序遍历的顺序构建一棵基于二叉链表存储结构的二叉树(如图所示),并对构建的二叉树实现先序、中序和后序遍历,并输出对应的遍历序列。 实验要求: 1、构建一个二叉链表存储结构的二叉树。 2、基于上述构建的二叉树进行先序、中序和后序遍历操作。用Java实现
时间: 2023-07-16 14:15:38 浏览: 99
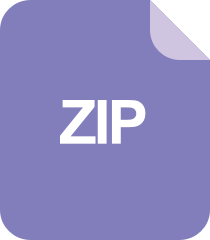
二叉树的几种操作,包括递归先序建立二叉树、先序遍历、中序遍历、后序遍历、非递归的各种遍历

以下是Java实现二叉树的构建和遍历操作的示例代码:
```java
class TreeNode {
int val;
TreeNode left;
TreeNode right;
public TreeNode(int val) {
this.val = val;
}
}
public class BinaryTreeTraversal {
// 构建二叉树
public static TreeNode buildTree(int[] preorder, int[] inorder) {
if (preorder == null || inorder == null || preorder.length == 0 || inorder.length == 0) {
return null;
}
return buildTree(preorder, inorder, 0, 0, inorder.length - 1);
}
private static TreeNode buildTree(int[] preorder, int[] inorder, int preIndex, int inStart, int inEnd) {
if (preIndex >= preorder.length || inStart > inEnd) {
return null;
}
TreeNode root = new TreeNode(preorder[preIndex]);
int inIndex = inStart;
while (inIndex <= inEnd) {
if (inorder[inIndex] == root.val) {
break;
}
inIndex++;
}
root.left = buildTree(preorder, inorder, preIndex + 1, inStart, inIndex - 1);
root.right = buildTree(preorder, inorder, preIndex + inIndex - inStart + 1, inIndex + 1, inEnd);
return root;
}
// 先序遍历
public static void preOrderTraversal(TreeNode root) {
if (root == null) {
return;
}
System.out.print(root.val + " ");
preOrderTraversal(root.left);
preOrderTraversal(root.right);
}
// 中序遍历
public static void inOrderTraversal(TreeNode root) {
if (root == null) {
return;
}
inOrderTraversal(root.left);
System.out.print(root.val + " ");
inOrderTraversal(root.right);
}
// 后序遍历
public static void postOrderTraversal(TreeNode root) {
if (root == null) {
return;
}
postOrderTraversal(root.left);
postOrderTraversal(root.right);
System.out.print(root.val + " ");
}
public static void main(String[] args) {
int[] preorder = {1, 2, 4, 5, 3, 6, 7};
int[] inorder = {4, 2, 5, 1, 6, 3, 7};
TreeNode root = buildTree(preorder, inorder);
System.out.print("先序遍历:");
preOrderTraversal(root);
System.out.print("\n中序遍历:");
inOrderTraversal(root);
System.out.print("\n后序遍历:");
postOrderTraversal(root);
}
}
```
输出结果为:
```
先序遍历:1 2 4 5 3 6 7
中序遍历:4 2 5 1 6 3 7
后序遍历:4 5 2 6 7 3 1
```
以上代码实现了二叉树的构建和先序、中序、后序遍历操作。其中,构建二叉树使用了递归方法,遍历操作同样使用了递归方法。这里给出的示例代码仅供参考,读者可以根据自己的需求进行修改。
阅读全文
相关推荐





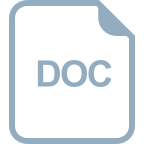
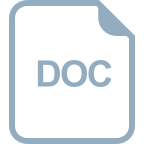
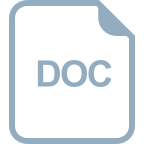







