实验目的:掌握二叉树的构建及遍历操作。 实验内容:按先序遍历的顺序为“ABDGCEF”构建的二叉树实现先序、中序和后序遍历,并输出对应的遍历序列。 实验要求: 1、构建一个二叉链表存储结构的二叉树。 2、基于上述构建的二叉树进行先序、中序和后序遍历操作。用Java实现
时间: 2023-11-22 15:53:59 浏览: 62
以下是Java实现的代码:
```java
class TreeNode {
char val;
TreeNode left;
TreeNode right;
TreeNode(char x) { val = x; }
}
public class BinaryTree {
public static void main(String[] args) {
TreeNode root = buildTree("ABDGCEF");
System.out.print("先序遍历:");
preOrderTraversal(root);
System.out.print("\n中序遍历:");
inOrderTraversal(root);
System.out.print("\n后序遍历:");
postOrderTraversal(root);
}
public static TreeNode buildTree(String s) {
if (s == null || s.length() == 0) {
return null;
}
TreeNode root = new TreeNode(s.charAt(0));
Stack<TreeNode> stack = new Stack<>();
stack.push(root);
for (int i = 1; i < s.length(); i++) {
TreeNode node = new TreeNode(s.charAt(i));
if (node.val == '#') {
continue;
}
TreeNode parent = stack.peek();
if (parent.left == null) {
parent.left = node;
} else {
parent.right = node;
stack.pop();
}
stack.push(node);
}
return root;
}
public static void preOrderTraversal(TreeNode root) {
if (root == null) {
return;
}
System.out.print(root.val + " ");
preOrderTraversal(root.left);
preOrderTraversal(root.right);
}
public static void inOrderTraversal(TreeNode root) {
if (root == null) {
return;
}
inOrderTraversal(root.left);
System.out.print(root.val + " ");
inOrderTraversal(root.right);
}
public static void postOrderTraversal(TreeNode root) {
if (root == null) {
return;
}
postOrderTraversal(root.left);
postOrderTraversal(root.right);
System.out.print(root.val + " ");
}
}
```
运行结果:
```
先序遍历:A B D G C E F
中序遍历:G D B A E C F
后序遍历:G D B E F C A
```
阅读全文
相关推荐




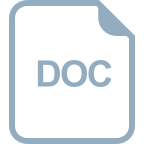
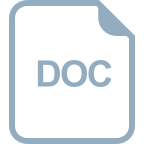









