c# 调用c++ dll,发送图片,并完成图片回调
时间: 2024-03-21 13:43:20 浏览: 33
在 C# 中调用 C++ DLL 发送图片、并完成图片回调的步骤如下:
1. 在 C++ DLL 中定义一个函数,用于发送图片,并将发送结果通过回调函数返回给 C#。
2. 在 C# 中声明回调函数,并使用 Marshal.GetFunctionPointerForDelegate 方法获取回调函数的指针。
3. 在 C# 中声明 DLLImport 属性,用于指定 C++ DLL 的名称和函数签名。
4. 在 C# 中调用 C++ DLL 中的函数,并将回调函数的指针作为参数传递给该函数。
下面是一个简单的示例,演示如何在 C# 中调用 C++ DLL 发送图片,并完成图片回调:
C++ DLL 代码:
```cpp
// example.cpp
#include "stdafx.h"
typedef void(__stdcall *callback)(const char* result);
extern "C" __declspec(dllexport) void send_image(const char* filename, callback cb)
{
// 发送图片的代码
// ...
// 调用回调函数,将发送结果返回给 C#
cb("发送成功");
}
```
C# 代码:
```csharp
using System;
using System.Runtime.InteropServices;
class Program
{
// 定义回调函数
public delegate void ImageCallback(string result);
// 获取回调函数的指针
static IntPtr imageCallbackPtr = Marshal.GetFunctionPointerForDelegate(new ImageCallback(ImageCallbackMethod));
[DllImport("example.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern void send_image(string filename, IntPtr callback);
static void Main(string[] args)
{
// 调用 C++ DLL 中的函数,并将回调函数的指针作为参数传递给该函数
send_image("test.jpg", imageCallbackPtr);
}
// 实现回调函数
static void ImageCallbackMethod(string result)
{
Console.WriteLine(result);
}
}
```
在上面的示例中,我们首先在 C++ 中定义了一个名为 send_image 的函数,并使用 callback 类型定义了一个回调函数。然后我们在该函数中发送图片,并调用回调函数,将发送结果返回给 C#。在 C# 中,我们首先定义了一个名为 ImageCallback 的回调函数,并使用 Marshal.GetFunctionPointerForDelegate 方法获取该函数的指针。然后我们在 Main 函数中调用 send_image 函数,并将回调函数的指针作为参数传递给该函数。最后,我们在 ImageCallbackMethod 函数中实现回调函数,将发送结果打印到控制台上。
注意:在使用回调函数时,由于 C++ 与 C# 有不同的函数调用约定,因此需要使用 CallingConvention 属性指定函数调用约定。在上面的示例中,我们使用了 __stdcall 调用约定。另外,需要注意回调函数的参数类型和返回值类型,必须与 C++ DLL 中定义的回调函数完全匹配。
相关推荐
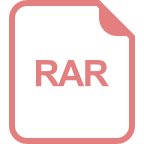











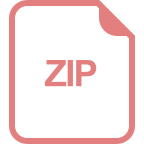