使用c++做一个坦克大战游戏
时间: 2023-12-25 15:29:53 浏览: 31
好的,下面是一个简单的坦克大战游戏实现的代码示例。这个示例实现了基本的游戏逻辑和图形界面,您可以在其基础上做出更多的改进和优化。
```
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <conio.h>
using namespace std;
const int WIDTH = 80; // 游戏界面宽度
const int HEIGHT = 25; // 游戏界面高度
class Tank {
public:
Tank(int x, int y, char ch) : x(x), y(y), ch(ch), dir(0), speed(1), bullets(10), power(1) {}
void move();
void fire();
void show();
bool hit(int px, int py);
friend class Game;
private:
int x;
int y;
char ch;
int dir;
int speed;
int bullets;
int power;
};
void Tank::move() {
if (_kbhit()) {
int c = _getch();
switch (c) {
case 'a':
dir = 0;
x -= speed;
break;
case 'd':
dir = 1;
x += speed;
break;
case 'w':
dir = 2;
y -= speed;
break;
case 's':
dir = 3;
y += speed;
break;
}
}
if (x < 0) x = 0;
if (x >= WIDTH) x = WIDTH - 1;
if (y < 0) y = 0;
if (y >= HEIGHT) y = HEIGHT - 1;
}
void Tank::fire() {
if (bullets <= 0) {
cout << "No bullets left!" << endl;
return;
}
bullets--;
switch (dir) {
case 0:
cout << "Bullet fired to left." << endl;
break;
case 1:
cout << "Bullet fired to right." << endl;
break;
case 2:
cout << "Bullet fired to up." << endl;
break;
case 3:
cout << "Bullet fired to down." << endl;
break;
}
}
void Tank::show() {
cout << ch;
}
bool Tank::hit(int px, int py) {
if (px == x && py == y) {
cout << "Tank hit!" << endl;
return true;
}
return false;
}
class Game {
public:
Game();
void start();
private:
Tank player;
Tank enemy;
};
Game::Game() : player(0, 0, 'P'), enemy(WIDTH - 1, HEIGHT - 1, 'E') {}
void Game::start() {
srand(time(NULL));
while (true) {
system("cls");
player.move();
player.show();
enemy.show();
if (enemy.hit(player.x, player.y)) {
cout << "You lose!" << endl;
break;
}
if (rand() % 100 < 10) {
enemy.fire();
}
if (player.hit(enemy.x, enemy.y)) {
cout << "You win!" << endl;
break;
}
Sleep(50);
}
}
int main() {
Game game;
game.start();
return 0;
}
```
这个示例实现了一个基本的坦克大战游戏,玩家可以使用键盘控制坦克移动和发射炮弹,敌方坦克会随机发射炮弹并追踪玩家坦克。当玩家坦克和敌方坦克碰撞时游戏结束,玩家需要尽可能地摧毁敌方坦克以获得胜利。
相关推荐
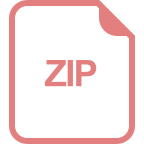
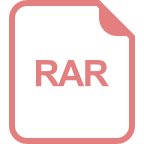












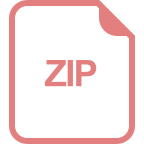
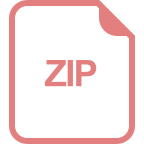
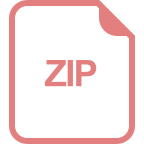