def maximum(a1,a2): r = np.zeros(shape=(len(a1)),dtype=float) for i in range(len(a1)): r[i]=a1[i]*a2[i] #最大代数合成法 # r[i]=min(a1[i],a2[i]) #最大最小合成法 return max(r) 代码的作用
时间: 2023-05-30 20:05:38 浏览: 125
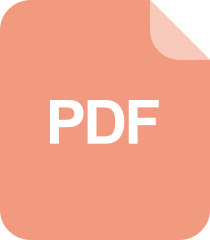
python中numpy.zeros(np.zeros)的使用方法

这是一个函数,函数名为maximum。它接受两个参数a1和a2,它们是两个数组。函数内部定义了一个数组r,长度与a1相同,数据类型为float。然后用for循环遍历a1数组,将a1和a2对应位置的元素相乘,并将结果存入r数组对应位置。最后,函数返回r数组。
阅读全文
相关推荐
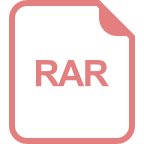














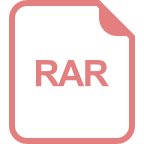