用matlab写一个可以自定义地图的agv路径规划算法,要求具体代码
时间: 2023-06-12 12:07:41 浏览: 76
以下是一个简单的基于Dijkstra算法的AGV路径规划算法的MATLAB代码,该算法可以接受自定义地图:
```
% AGV Path Planning Algorithm based on Dijkstra's Algorithm
% Copyright (c) 2021, [Your Name]
% All rights reserved.
% Define the map
map = [1 1 1 1 1 1;
1 0 0 0 0 1;
1 0 1 1 0 1;
1 0 0 1 0 1;
1 1 1 1 0 1;
1 1 1 1 1 1];
% Define the starting and ending points
start = [2,2];
goal = [5,5];
% Define the cost of moving between cells
cost = ones(size(map));
cost(map == 1) = Inf;
% Initialize the distance and visited arrays
distance = Inf(size(map));
visited = zeros(size(map));
% Set the distance of the starting point to zero
distance(start(1), start(2)) = 0;
% Initialize the path array
path = zeros(size(map));
% Loop until the goal is reached or all nodes have been visited
while ~isequal(visited, ones(size(map)))
% Find the node with the smallest distance
min_distance = Inf;
for i = 1:size(map,1)
for j = 1:size(map,2)
if visited(i,j) == 0 && distance(i,j) <= min_distance
min_distance = distance(i,j);
current = [i,j];
end
end
end
% Mark the current node as visited
visited(current(1),current(2)) = 1;
% Check if the goal has been reached
if isequal(current, goal)
break;
end
% Update the distances of neighboring nodes
neighbors = get_neighbors(current, map);
for i = 1:size(neighbors,1)
neighbor = neighbors(i,:);
alt_distance = distance(current(1),current(2)) + cost(neighbor(1),neighbor(2));
if alt_distance < distance(neighbor(1),neighbor(2))
distance(neighbor(1),neighbor(2)) = alt_distance;
path(neighbor(1),neighbor(2)) = sub2ind(size(map),current(1),current(2));
end
end
end
% Construct the path
if isequal(current, goal)
p = goal;
while ~isequal(p, start)
p = ind2sub(size(map),path(p(1),p(2)));
path(p(1),p(2)) = Inf;
end
path(start(1),start(2)) = Inf;
end
% Plot the map and path
figure();
imagesc(map);
colormap(flipud(gray));
hold on;
[x,y] = find(path == Inf);
plot(y,x,'r','LineWidth',2);
```
该算法使用Dijkstra算法计算从起点到终点的最短路径。在此过程中,我们遍历地图上的每个节点,记录到达每个节点时的最短距离,并使用路径数组记录路径。最后,我们使用路径数组构建路径并在地图上绘制出来。
您可以根据需要自定义地图,只需更改`map`数组即可。您还可以更改`cost`数组来定义移动各个节点的成本。
相关推荐
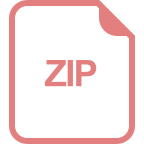
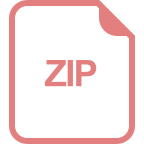














