封装函数,帮我解析这段网页里面的数据,最后告诉我具体实现的步骤:<h3 class="tb-main-title" data-title="ROG枪神7Plus 超竞版 13代酷睿i9 电竞游戏本4070 4080笔记本电脑"> ROG枪神7Plus 超竞版 13代酷睿i9 电竞游戏本4070 4080笔记本电脑 </h3>
时间: 2024-01-09 16:03:56 浏览: 64
可以使用 Python 中的 Beautiful Soup 库来解析网页并提取所需数据。
实现步骤如下:
1. 安装 Beautiful Soup 库,可以使用 pip install beautifulsoup4 命令进行安装。
2. 导入库,创建 Beautiful Soup 对象并读取网页内容:
```python
from bs4 import BeautifulSoup
import requests
url = 'https://www.example.com' # 替换为需要解析的网页
html = requests.get(url).text
soup = BeautifulSoup(html, 'html.parser')
```
3. 找到包含目标数据的标签,可以使用标签名、类名、属性等方式进行查找,例如:
```python
h3_tag = soup.find('h3', class_='tb-main-title')
```
这里使用了 `find()` 方法,返回第一个符合条件的标签。`class_` 参数用于指定 class 属性值。
4. 提取标签中的数据:
```python
data_title = h3_tag['data-title']
```
这里使用了字典形式的访问方式,获取了标签中 `data-title` 属性的值。
5. 将上述代码封装成函数:
```python
from bs4 import BeautifulSoup
import requests
def parse_data_title(url):
html = requests.get(url).text
soup = BeautifulSoup(html, 'html.parser')
h3_tag = soup.find('h3', class_='tb-main-title')
data_title = h3_tag['data-title']
return data_title
```
这个函数接收一个 URL 参数,返回解析后得到的数据。
阅读全文
相关推荐
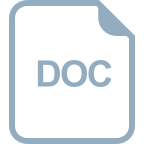
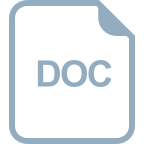
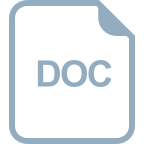
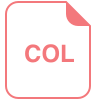
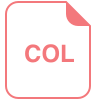
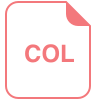
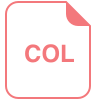




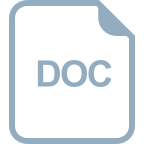
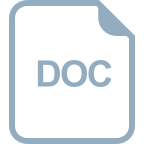
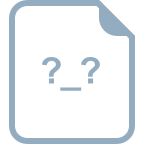
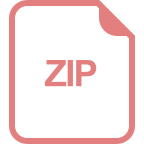
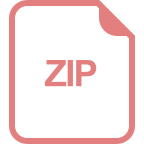
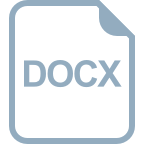