cv2.boundingRect()用法
时间: 2024-02-06 16:03:54 浏览: 30
`cv2.boundingRect()` 是 OpenCV 库中的一个函数,用于计算轮廓的边界矩形。该函数可以接收一个轮廓作为输入,然后返回一个矩形,该矩形紧密地包围轮廓的边缘,并返回矩形的左上角坐标和宽度、高度。
函数原型如下:
```python
x, y, w, h = cv2.boundingRect(contour)
```
其中,`contour` 是输入的轮廓,`x` 和 `y` 表示矩形的左上角坐标,`w` 和 `h` 表示矩形的宽度和高度。
使用 `cv2.boundingRect()` 函数可以很方便地将轮廓包围在一个矩形中,从而进行一些后续的操作,例如图像裁剪、对象检测等。
相关问题
cv2.boundingRect的用法
cv2.boundingRect是OpenCV库中用于计算轮廓的边界框的函数。它可以根据给定的轮廓点集计算出包围该轮廓的矩形框。
该函数的语法如下:
x, y, w, h = cv2.boundingRect(contour)
其中,contour是输入的轮廓点集,x和y是边界框的左上角坐标,w和h分别是边界框的宽度和高度。
具体使用方法如下:
1. 首先,使用cv2.findContours函数找到图像中的轮廓点集。
2. 针对每个轮廓,使用cv2.boundingRect函数计算其边界框。
3. 获取边界框的左上角坐标(x, y)和宽度w以及高度h。
例如,假设我们有一个轮廓点集contour,我们可以使用boundingRect函数计算出该轮廓的边界框:
```python
import cv2
# 假设contour是一个轮廓点集
contour = np.array([[10, 10], [20, 10], [20, 20], [10, 20]])
# 计算边界框
x, y, w, h = cv2.boundingRect(contour)
# 打印结果
print("边界框左上角坐标:({}, {})".format(x, y))
print("边界框宽度:{}".format(w))
print("边界框高度:{}".format(h))
```
输出结果如下:
```
边界框左上角坐标:(10, 10)
边界框宽度:10
边界框高度:10
```
上述代码中,我们假设contour是一个包含四个点的轮廓点集,通过调用boundingRect函数计算出该轮廓的边界框。然后,我们打印出边界框的左上角坐标、宽度和高度。
cv2.boundingRect()是怎么用的
cv2.boundingRect()是一个OpenCV函数,用于计算给定轮廓的边界矩形。它的语法如下:
```python
x, y, w, h = cv2.boundingRect(contour)
```
其中,`contour`是输入的轮廓,`x`和`y`是矩形左上角的坐标,`w`和`h`是矩形的宽度和高度。
这个函数的作用是帮助我们计算一个图像区域的最小矩形边界框,可以用于图像分割、目标检测等任务。使用方法如下:
```python
import cv2
# 读取图像并转换为灰度图像
img = cv2.imread('image.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化处理
_, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 查找图像轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 遍历轮廓并计算边界矩形
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
# 显示结果
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码中,我们首先读取图像并将其转换为灰度图像,然后对其进行二值化处理。接着,我们使用`cv2.findContours()`函数查找图像中的轮廓,然后遍历每个轮廓并计算其边界矩形,最后在图像上绘制矩形框并显示结果。
相关推荐
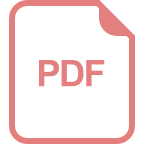
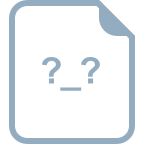
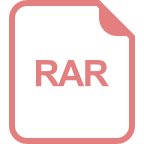













