Cesium fragmentShaderSource 代码
时间: 2024-05-07 13:15:52 浏览: 125
以下是一个基本的Cesium fragmentShaderSource样例:
```
varying vec2 v_textureCoordinates;
uniform sampler2D u_texture;
void main() {
gl_FragColor = texture2D(u_texture, v_textureCoordinates);
}
```
这个着色器代码片段描述了如何在Cesium中呈现纹理。它使用一个2D纹理采样器,将纹理图像映射到当前的片段上。在这个着色器中,我们声明了一个varying变量v_textureCoordinates,它接收从顶点着色器传递下来的纹理坐标。我们还声明了一个uniform变量u_texture,它表示我们要绑定的纹理对象。在Main函数中,我们使用texture2D函数从纹理中提取颜色值,并将其分配给当前的片段(gl_FragColor)。
相关问题
cesium自定义渲染代码
在Cesium中,您可以使用`CustomDataSource`和`CustomRenderPrimitive`来自定义渲染代码。
`CustomDataSource`是一种特殊类型的`DataSource`,它允许您在场景中添加自定义的实体和几何图形。您可以使用`CustomRenderPrimitive`在场景中添加自定义的渲染图元,每个渲染图元都可以实现自己的渲染逻辑。
以下是一个简单的示例,演示如何使用`CustomDataSource`和`CustomRenderPrimitive`来自定义渲染代码:
```javascript
// 创建Cesium Viewer
var viewer = new Cesium.Viewer('cesiumContainer');
// 创建自定义数据源
var customDataSource = new Cesium.CustomDataSource('custom');
// 添加自定义实体
var entity = customDataSource.entities.add({
position: Cesium.Cartesian3.fromDegrees(-75.59777, 40.03883),
billboard: {
image: 'path/to/image.png',
scale: 2.0
}
});
viewer.dataSources.add(customDataSource);
// 创建自定义渲染图元
var customPrimitive = new Cesium.CustomRenderPrimitive({
geometryInstances: new Cesium.GeometryInstance({
geometry: new Cesium.RectangleGeometry({
rectangle: Cesium.Rectangle.fromDegrees(-75.0, 35.0, -125.0, 45.0)
}),
attributes: {
color: Cesium.ColorGeometryInstanceAttribute.fromColor(Cesium.Color.RED)
}
}),
fragmentShaderSource: 'void main() { gl_FragColor = vec4(1.0, 0.0, 0.0, 1.0); }',
vertexShaderSource: 'attribute vec3 position3DHigh;\
attribute vec3 position3DLow;\
attribute vec4 color;\
varying vec4 v_color;\
void main() {\
vec4 position = czm_computePosition();\
gl_Position = czm_viewProjection * position;\
v_color = color;\
}',
renderState: {
blending: Cesium.BlendingState.DISABLED,
depthTest: {
enabled: true,
func: Cesium.DepthFunction.LESS
},
depthMask: true,
polygonOffset: {
enabled: false,
factor: 0.0,
units: 0.0
}
}
});
viewer.scene.primitives.add(customPrimitive);
```
在上面的代码中,我们首先创建了一个Cesium Viewer,并创建了一个自定义数据源。然后,我们向自定义数据源中添加了一个自定义实体,并将其添加到场景中。接下来,我们创建了一个自定义渲染图元,并将其添加到场景中。在自定义渲染图元中,我们使用了自定义的顶点着色器和片段着色器,并设置了渲染状态。
请注意,上面的示例仅用于演示目的。实际应用中,您可能需要更复杂的渲染逻辑和着色器代码。
Cesium DrawCommand 怎么裁剪 给个代码
好的,下面是一个简单的示例代码,演示如何在Cesium的DrawCommand中使用裁剪平面:
```javascript
// 定义裁剪平面
var plane = Cesium.Plane.fromPointNormal(new Cesium.Cartesian3(0, 0, 0), new Cesium.Cartesian3(0, 0, 1));
// 创建DrawCommand
var drawCommand = new Cesium.DrawCommand({
// ...
// 在渲染前启用裁剪平面
// 注意:webgl中有多个裁剪平面,这里只启用了第一个(即CLIP_DISTANCE0)
// 如果需要使用多个裁剪平面,可以使用gl.enableVertexAttribArray()启用其他裁剪平面
// 参考:https://developer.mozilla.org/en-US/docs/Web/API/WebGLRenderingContext/enableVertexAttribArray
vertexShaderSource : 'attribute vec4 position;\n' +
'uniform mat4 modelViewProjection;\n' +
'uniform vec4 plane;\n' +
'varying float distance;\n' +
'void main()\n' +
'{\n' +
' gl_ClipDistance[0] = dot(modelViewProjection * position, plane);\n' +
' distance = gl_ClipDistance[0];\n' +
' gl_Position = modelViewProjection * position;\n' +
'}',
// 在片元着色器中检查裁剪平面
fragmentShaderSource : 'varying float distance;\n' +
'void main()\n' +
'{\n' +
' if (distance < 0.0) discard;\n' +
' gl_FragColor = vec4(1.0, 0.0, 0.0, 1.0);\n' +
'}',
// 传递裁剪平面的参数
uniformMap : {
plane : function() {
return plane;
}
}
});
// 渲染场景
var scene = viewer.scene;
scene.render();
// 清除裁剪平面
// 在渲染后,需要手动禁用裁剪平面,避免影响下一次渲染
var gl = scene.context._gl;
gl.disable(gl.CLIP_DISTANCE0);
```
这段代码中,我们在顶点着色器中计算了每个顶点到裁剪平面的距离,并将该距离存储到gl_ClipDistance数组中。然后,在片元着色器中,我们检查gl_ClipDistance数组的值,如果距离小于0,则丢弃该片元。最后,我们使用uniformMap将裁剪平面传递给DrawCommand。
需要注意的是,在渲染后,我们需要手动禁用裁剪平面,避免影响下一次渲染。
阅读全文
相关推荐
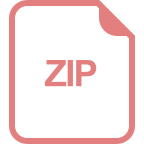
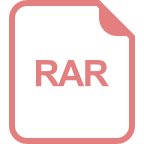










