python中优化一组自变量参数,使得目标函数达到最小值的办法有哪些?
时间: 2024-02-24 21:54:56 浏览: 27
Python中优化一组自变量参数使得目标函数达到最小值的方法有很多,以下是几种常用的方法:
1. Scipy.optimize模块:Scipy是Python中科学计算的核心库之一,其中的optimize模块提供了多种优化算法,包括Nelder-Mead、BFGS、L-BFGS-B、Powell等。可以使用这些算法进行函数最小化,可以处理约束问题。例如:
```python
from scipy.optimize import minimize
def my_func(x):
return x[0]**2 + x[1]**2
res = minimize(my_func, [0, 0])
print(res.x)
```
这里使用了Nelder-Mead算法,将函数my_func最小化,并返回最小值的自变量值。
2. Differential Evolution(DE)算法:DE是一种全局优化算法,用于寻找多维非线性函数的最小值。它是一种进化算法,可以避免局部最优解。可以使用Python库DEAP实现DE算法,例如:
```python
from deap import algorithms, base, creator, tools
def my_func(x):
return x[0]**2 + x[1]**2
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
creator.create("Individual", list, fitness=creator.FitnessMin)
toolbox = base.Toolbox()
toolbox.register("attr_float", random.uniform, -10, 10)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_float, n=2)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("evaluate", my_func)
toolbox.register("mate", tools.cxSimulatedBinaryBounded, low=-10, up=10, eta=20.0)
toolbox.register("mutate", tools.mutPolynomialBounded, low=-10, up=10, eta=20.0, indpb=1.0/2)
toolbox.register("select", tools.selRandom)
pop = toolbox.population(n=100)
result, logbook = algorithms.eaSimple(pop, toolbox, cxpb=0.5, mutpb=0.2, ngen=50, verbose=False)
print(result)
```
这里使用DEAP实现DE算法,将函数my_func最小化,并返回最小值的自变量值。
3. 遗传算法(Genetic Algorithm,GA):GA是一种基于生物进化原理的优化算法,可以用于优化多个自变量的函数。可以使用Python库DEAP实现GA算法,例如:
```python
from deap import algorithms, base, creator, tools
def my_func(x):
return x[0]**2 + x[1]**2
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
creator.create("Individual", list, fitness=creator.FitnessMin)
toolbox = base.Toolbox()
toolbox.register("attr_float", random.uniform, -10, 10)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_float, n=2)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("evaluate", my_func)
toolbox.register("mate", tools.cxBlend, alpha=0.5)
toolbox.register("mutate", tools.mutGaussian, mu=0, sigma=0.1, indpb=0.05)
toolbox.register("select", tools.selTournament, tournsize=3)
pop = toolbox.population(n=100)
result, logbook = algorithms.eaSimple(pop, toolbox, cxpb=0.5, mutpb=0.2, ngen=50, verbose=False)
print(result)
```
这里使用DEAP实现GA算法,将函数my_func最小化,并返回最小值的自变量值。
以上是一些常用的Python优化算法,还有其他的优化算法也可以使用,例如遗传编程、粒子群算法等。选择哪种算法取决于具体的问题和数据。
相关推荐
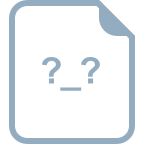
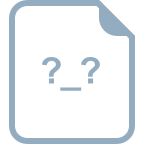














