二分K均值实现图片分割
时间: 2023-09-05 10:11:16 浏览: 37
二分K均值是一种基于K均值算法的改进方法,用于图像分割时可以得到更好的结果。
实现步骤如下:
1. 将图像转化为一维数组。
2. 设定初始的K个聚类中心(可以随机选择或使用其他方法),计算每个像素点与聚类中心的距离,并将其归入距离最近的类别中。
3. 计算每个类别的平均值作为新的聚类中心。
4. 重复步骤2和步骤3,直到聚类中心不再发生变化或达到设定的迭代次数。
5. 对于二分K均值,将所有样本归入一个初始类别中,然后将其分成两个子类别。对每一个子类别重复1-4步骤,直到达到设定的最大类别数或某些停止条件。
6. 最终将每个像素点归入距离最近的聚类中心所在的类别中,即可得到图像分割结果。
下面是Python代码实现:
```python
import numpy as np
from PIL import Image
def binary_kmeans(image_path, k_max=10, max_iter=20):
# Load image
image = Image.open(image_path)
pixels = np.array(image).reshape(-1, 3)
# Initialize with one cluster
clusters = [(0, pixels)]
# Binary K-means loop
while len(clusters) < k_max:
# Choose the cluster with maximum SSE
sse_list = [np.sum((cluster[1] - np.mean(cluster[1], axis=0)) ** 2)
for cluster in clusters]
max_sse_idx = np.argmax(sse_list)
max_sse_cluster = clusters[max_sse_idx]
# Split the cluster into two sub-clusters
kmeans = KMeans(n_clusters=2, max_iter=max_iter).fit(max_sse_cluster[1])
sub_clusters = [(max_sse_cluster[0] * 2 + i, max_sse_cluster[1][kmeans.labels_ == i])
for i in range(2)]
# Replace the original cluster with the sub-clusters
clusters = clusters[:max_sse_idx] + sub_clusters + clusters[max_sse_idx + 1:]
# Assign each pixel to the closest cluster
labels = np.zeros(len(pixels), dtype=int)
for cluster in clusters:
labels[np.argmin(np.sum((pixels - np.mean(cluster[1], axis=0)) ** 2, axis=1))] = cluster[0]
# Reshape the labels back to an image
return labels.reshape(image.size[1], image.size[0])
```
其中,`image_path`为需要分割的图像路径,`k_max`为最大聚类数,`max_iter`为每个子类别的最大迭代次数。该函数返回每个像素点所属的类别标签,可以使用不同的颜色来可视化分割结果。
相关推荐
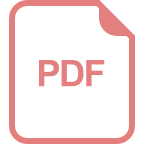
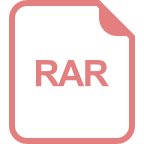
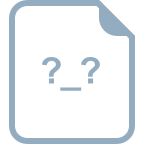














