void countEducation() { map<string, map<string, int>> departmentCount; Node* p = head->next; while (p != nullptr) { string department = p->data.getDepartment(); string education = p->data.getEducation(); departmentCount[department][education]++; p = p->next; } for (auto it1 : departmentCount) { cout << it1.first << endl; for (auto it2 : it1.second) { cout << " " << it2.first << ":" << it2.second << endl; } } }这段代码应该如何优化改进?
时间: 2024-03-07 13:48:31 浏览: 260
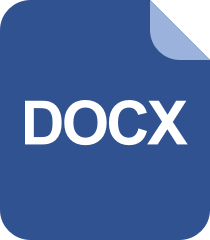
八种常见数据结构介绍和C++案例.docx
这段代码可以考虑使用引用类型来避免不必要的复制操作,同时可以使用auto关键字来简化迭代器的声明。
代码示例:
```cpp
void countEducation() {
map<string, map<string, int>> departmentCount;
Node* p = head->next;
while (p != nullptr) {
string& department = p->data.getDepartment();
string& education = p->data.getEducation();
departmentCount[department][education]++;
p = p->next;
}
for (auto& [department, educationCount] : departmentCount) {
cout << department << endl;
for (auto& [education, count] : educationCount) {
cout << " " << education << ":" << count << endl;
}
}
}
```
另外,可以在Node类中添加访问器函数,使得数据的访问更加安全和直观。例如:
```cpp
class Node {
public:
Teacher& getData() { return data; }
Node* getNext() { return next; }
void setNext(Node* node) { next = node; }
private:
Teacher data;
Node* next;
};
```
这样,在遍历链表时,可以使用p->getData().getDepartment()和p->getData().getEducation()来访问数据。
阅读全文
相关推荐
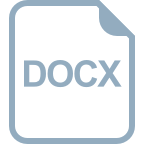
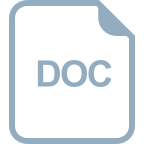







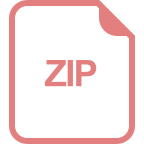
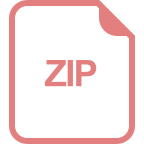
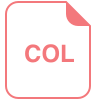
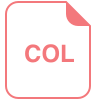




