下面程序可以找出两个整数的较大数。 #include <iostream> using namespace std; _____________________; //函数声明 int main( ) { int a ,b ,Max; cout<< " Please input two integer numbers: "; cin>>a>>b; Max = ; //函数调用 cout<< " The Large number: " << Max <<endl; return 0; } int Max_int(int x , int y) { ; }//函数返回结果
时间: 2024-02-16 10:06:43 浏览: 93
下面是完整的程序,包括函数定义和函数调用:
```
#include <iostream>
using namespace std;
int Max_int(int x, int y); //函数声明
int main() {
int a, b, Max;
cout << "Please input two integer numbers: ";
cin >> a >> b;
Max = Max_int(a, b); //函数调用
cout << "The Large number: " << Max << endl;
return 0;
}
int Max_int(int x, int y) { //函数定义
if (x > y) {
return x;
} else {
return y;
}
}
```
这个程序通过调用 `Max_int` 函数来找出两个整数的较大数。函数定义中,如果 `x` 大于 `y`,则返回 `x`,否则返回 `y`。在主函数中,先读入两个整数 `a` 和 `b`,然后调用 `Max_int` 函数来找出它们的较大数,并将结果赋给变量 `Max`,最后输出结果。
相关问题
#include<iostream> using namespace std; int main()
这是一个使用C++语言编写的控制台程序,它包含了头文件<iostream>,并使用了命名空间std。程序的主函数为int main(),其中定义了三个变量n、i和k,并从标准输入流中读取一个整数赋值给n。接着使用for循环从n*n开始倒序遍历到1,每次输出一个整数并使用setw(5)进行格式化输出,同时将计数器k加1,当k能被n整除时输出换行符。最后输出一个换行符并返回0表示程序正常结束。
#include<iostream> #include<random> using namespace std; int main(){ int a = random(1,100); cout<<a; }
这个C++代码片段尝试使用`std`命名空间,并从头文件`#include <iostream>`和`#include <random>`导入必要的库。但是,实际上在C++11标准及以后版本中,`random()`函数已经不再直接存在于`std`命名空间内,而是应该使用`std::mt19937`等随机数引擎配合`std::uniform_int_distribution`来生成随机数。
正确的写法应该是:
```cpp
#include <iostream>
#include <random>
using namespace std;
int main() {
// 使用Mersenne Twister算法作为随机数引擎
mt19937 engine; // 需要先实例化一个随机数引擎
// 设定随机数的范围
uniform_int_distribution<int> dist(1, 100); // 注意是int而不是random
// 生成随机数并输出
int a = dist(engine);
cout << "Random number between 1 and 100: " << a << endl;
return 0;
}
```
在上述代码中,`dist(engine)`会生成1到100之间的随机整数。
阅读全文
相关推荐
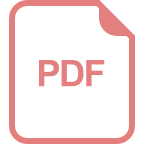
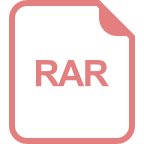













