【问题描述】设二叉树按二叉链表存放,写算法判别一棵二叉树是否是一棵正则二叉树。正则二叉树是指在二叉树中不存在子树个数为1的结点。 【输入说明】二叉树的前序和中序遍历序列,以此构造二叉树的链式存储 【输出说明】若该二叉树是一棵正则二叉树,则输出Yes,否则输出No 【样例输入】 ABHDCEFG HBDAEFCG 【样例输出】 No
时间: 2023-12-02 08:05:47 浏览: 50
以下是 Python 代码实现:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def build_tree(preorder, inorder):
if not preorder or not inorder:
return None
root_val = preorder[0]
root = TreeNode(root_val)
idx = inorder.index(root_val)
root.left = build_tree(preorder[1:1+idx], inorder[:idx])
root.right = build_tree(preorder[1+idx:], inorder[idx+1:])
return root
def is_regular_tree(root):
if not root:
return True
if root.left and not root.right:
return False
if not root.left and root.right:
return False
return is_regular_tree(root.left) and is_regular_tree(root.right)
if __name__ == '__main__':
preorder = list(input().strip())
inorder = list(input().strip())
root = build_tree(preorder, inorder)
if is_regular_tree(root):
print('Yes')
else:
print('No')
```
思路解析:
1. 根据前序遍历和中序遍历构造二叉树;
2. 遍历每个节点,若存在子树个数为1的节点,则不是正则二叉树;
3. 若所有节点都满足条件,则是正则二叉树。
时间复杂度:$O(n^2)$,其中 $n$ 是二叉树中节点个数。因为要遍历每个节点,每个节点需要遍历其左右子树,所以总时间复杂度为 $n+n-1+n-2+...+1=n(n+1)/2=O(n^2)$。
阅读全文
相关推荐












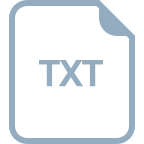
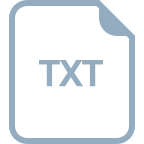