用C++从调用depthimage_to_laserscan功能包将景深图转为激光图
时间: 2024-03-02 12:50:41 浏览: 29
好的,我会尽力回答你的问题。
首先,depthimage_to_laserscan是ROS(机器人操作系统)的一个功能包,用于将深度图像转换为激光雷达数据。如果你想使用C++调用这个功能包,需要先在ROS环境下安装depthimage_to_laserscan功能包,然后使用ROS的C++ API来编写程序。
以下是一个简单的C++程序示例,用于将深度图像转换为激光雷达数据:
```cpp
#include <ros/ros.h>
#include <sensor_msgs/Image.h>
#include <sensor_msgs/LaserScan.h>
#include <depthimage_to_laserscan/DepthImageToLaserScan.h>
int main(int argc, char** argv)
{
// 初始化ROS节点
ros::init(argc, argv, "depth_to_laser");
// 创建ROS节点句柄
ros::NodeHandle nh;
// 创建DepthImageToLaserScan客户端,用于调用depthimage_to_laserscan服务
ros::ServiceClient client = nh.serviceClient<depthimage_to_laserscan::DepthImageToLaserScan>("depthimage_to_laserscan");
// 创建激光雷达数据发布者
ros::Publisher laser_pub = nh.advertise<sensor_msgs::LaserScan>("laser_scan", 1);
// 创建深度图像订阅者
ros::Subscriber depth_sub = nh.subscribe<sensor_msgs::Image>("depth_image", 1, [&](const sensor_msgs::Image::ConstPtr& depth_msg){
// 创建depthimage_to_laserscan服务请求
depthimage_to_laserscan::DepthImageToLaserScan srv;
srv.request.image = *depth_msg;
// 调用depthimage_to_laserscan服务,将深度图像转换为激光雷达数据
if (client.call(srv))
{
// 将激光雷达数据发布出去
laser_pub.publish(srv.response.scan);
}
else
{
ROS_ERROR("Failed to call depthimage_to_laserscan service");
}
});
// 进入ROS事件循环
ros::spin();
return 0;
}
```
这个程序中,我们使用了ROS的C++ API来创建了一个DepthImageToLaserScan客户端,用于调用depthimage_to_laserscan服务。然后,我们创建了一个激光雷达数据发布者和一个深度图像订阅者。当接收到深度图像消息时,我们就创建了一个depthimage_to_laserscan服务请求,将深度图像转换为激光雷达数据,并将激光雷达数据发布出去。
当你运行这个程序时,记得要先启动ROS环境和depthimage_to_laserscan节点,然后再运行这个程序。
相关推荐
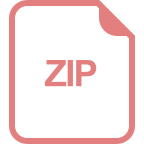
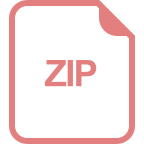














