用cpp判断一个字符串数组是否是回文数组
时间: 2023-04-02 14:02:55 浏览: 126
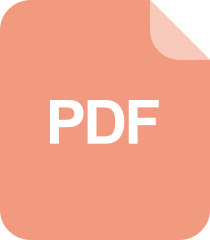
算法笔记_12.16-12.22
可以使用双指针法来判断一个字符串数组是否是回文数组。具体实现如下:
bool isPalindrome(char* s) {
int len = strlen(s);
int left = , right = len - 1;
while (left < right) {
if (s[left] != s[right]) {
return false;
}
left++;
right--;
}
return true;
}
其中,s 表示字符串数组,len 表示字符串数组的长度,left 和 right 分别表示左右指针的位置。在 while 循环中,如果左右指针所指向的字符不相等,则说明该字符串数组不是回文数组,返回 false;否则,左右指针向中间移动,继续比较。如果最终左右指针相遇,则说明该字符串数组是回文数组,返回 true。
阅读全文
相关推荐
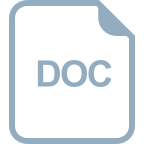
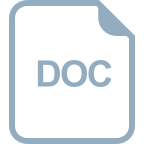






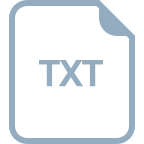
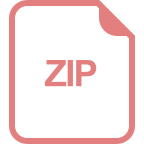
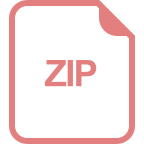


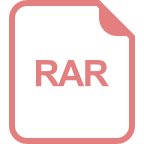
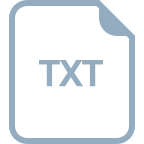
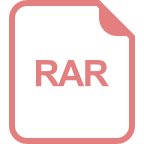

