图卷积神经网络要求每一层之间都加入Res残差连接,要求有12层图卷积层,并且完成对cora数据集的训练与测试,写一段python代码
时间: 2024-04-30 12:21:58 浏览: 21
好的,下面是一份使用PyTorch实现12层ResGCN对cora数据集进行训练和测试的代码:
首先,需要引入必要的库和包:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch_geometric.nn import GCNConv
from torch_geometric.datasets import Planetoid
```
然后,定义ResGCN模型,包括ResGCN层和一个全连接层:
```python
class ResGCN(nn.Module):
def __init__(self, in_channels, out_channels):
super(ResGCN, self).__init__()
self.conv1 = GCNConv(in_channels, out_channels)
self.conv2 = GCNConv(out_channels, out_channels)
self.res_conv = GCNConv(in_channels, out_channels)
self.fc = nn.Linear(out_channels, 7)
def forward(self, x, edge_index):
res = self.res_conv(x, edge_index)
x = self.conv1(x, edge_index)
x = F.relu(x)
x = self.conv2(x, edge_index)
x = x + res
x = F.relu(x)
x = self.fc(x)
return F.log_softmax(x, dim=1)
```
接着,加载cora数据集:
```python
dataset = Planetoid(root='/tmp/Cora', name='Cora')
data = dataset[0]
```
然后,定义模型和优化器:
```python
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = ResGCN(dataset.num_features, 64).to(device)
optimizer = torch.optim.Adam(model.parameters(), lr=0.01, weight_decay=5e-4)
```
接下来,定义训练函数和测试函数:
```python
def train():
model.train()
optimizer.zero_grad()
out = model(data.x.to(device), data.edge_index.to(device))
loss = F.nll_loss(out[data.train_mask], data.y[data.train_mask].to(device))
loss.backward()
optimizer.step()
return loss.item()
def test():
model.eval()
out = model(data.x.to(device), data.edge_index.to(device))
pred = out.argmax(dim=1)
test_correct = pred[data.test_mask] == data.y[data.test_mask].to(device)
test_acc = int(test_correct.sum()) / int(data.test_mask.sum())
return test_acc
```
最后,进行训练和测试,并输出测试准确率:
```python
for epoch in range(1, 101):
loss = train()
test_acc = test()
print('Epoch: {:03d}, Loss: {:.4f}, Test Acc: {:.4f}'.format(epoch, loss, test_acc))
```
完整代码如下:
相关推荐
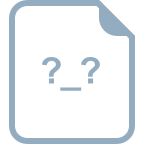
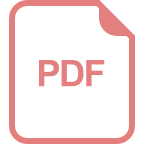
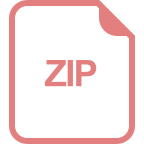














