用C++求e^x。描述:编写程序,输入x,根据公式e∧x=1+ x+ x^2/2+ x^3/6.....计算并输出的近似值,要求累加和到某项小于-6时为止(不要使用pow函数)。
时间: 2024-05-01 22:19:40 浏览: 6
可以使用以下代码来求e^x的近似值:
```
#include <stdio.h>
int main() {
double x, term = 1, result = 1;
int i = 1;
printf("Enter the value of x: ");
scanf("%lf", &x);
while (term >= -1e-6) {
term *= x / i;
result += term;
i++;
}
printf("e^%.2f = %.6f", x, result);
return 0;
}
```
程序中定义了变量x表示输入的x值,term表示当前项的值,result表示累加和的结果,i表示当前项的分母。
程序通过while循环不断计算每一项的值,当某一项小于-1e-6时停止计算。在循环中,首先计算当前项的值,然后将其加到结果中,然后更新分母i。
最后,程序输出e^x的近似值。注意,程序中没有使用pow函数,而是直接用x/i来计算每一项的值。
相关问题
用c++的math库求表达式: 1/2(a+1)x + 1/8
以下是用C++的math库求解表达式1/2(a+1)x + 1/8的示例代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main()
{
double a, x, result;
cout << "请输入a和x的值:" << endl;
cin >> a >> x;
result = 0.5 * (a + 1) * x + 0.125;
cout << "结果为:" << result << endl;
return 0;
}
```
其中,0.5表示1/2,0.125表示1/8。在计算过程中,先将(a+1)除以2,再乘以x,最后加上1/8,即可得到表达式的结果。
c++中输入整数x(x>=0)求e^x=1+x+x^2/2的阶乘一直到x^n/n的阶乘 的计算结果
这个问题涉及数学计算,可以直接回答。根据题意,可以使用循环计算每一项的阶乘,然后求和即可得到答案。以下是示例代码:
```c
#include <stdio.h>
int main() {
int x;
printf("请输入整数x:");
scanf("%d", &x);
double result = 1.0, term = 1.0;
int i, j;
for (i = 1; i <= x; i++) {
term *= x;
for (j = 2; j <= i; j++) {
term /= j;
}
result += term;
}
printf("e^x = %lf\n", result);
return 0;
}
```
当然,这只是一个简单的示例,实际应用中可能需要考虑数值精度等问题。
相关推荐
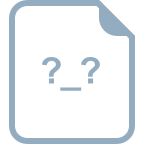
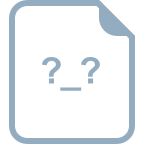












