用c++实现DES加密程序,要求可以输入明文,输出加密后的密文,输出生成的密钥,输出解密后的明文,用库函数写
时间: 2024-02-13 21:06:19 浏览: 192
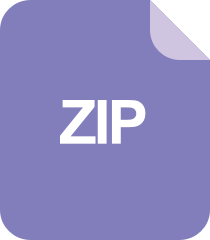
用c++编写DES加密
好的,我可以为您提供一个基于 OpenSSL 库的 C++ 实现 DES 加密的程序。请确认您已经在您的计算机上安装了 OpenSSL 库。
以下是代码:
```cpp
#include <iostream>
#include <iomanip>
#include <cstring>
#include <openssl/des.h>
using namespace std;
// 将字符串转成 64 位二进制数
void stringToBinary(const char *str, unsigned char *binary)
{
int len = strlen(str);
for (int i = 0; i < len; i++)
{
for (int j = 0; j < 8; j++)
{
binary[i * 8 + j] = (str[i] & (1 << (7 - j))) ? 1 : 0;
}
}
}
// 将 64 位二进制数转成字符串
void binaryToString(const unsigned char *binary, char *str)
{
for (int i = 0; i < 8; i++)
{
str[i] = 0;
for (int j = 0; j < 8; j++)
{
str[i] |= (binary[i * 8 + j] << (7 - j));
}
}
}
// 打印二进制数据
void printBinary(const unsigned char *binary, int len)
{
for (int i = 0; i < len; i++)
{
cout << setw(2) << setfill('0') << hex << (int)binary[i];
}
cout << endl;
}
int main()
{
// 明文
const char *plaintext = "Hello, DES!";
// 密钥
const char *key = "01234567";
// 明文长度
int plaintext_len = strlen(plaintext);
// 密钥长度
int key_len = strlen(key);
// 将明文和密钥转成 64 位二进制数
unsigned char plaintext_binary[plaintext_len * 8];
unsigned char key_binary[key_len * 8];
stringToBinary(plaintext, plaintext_binary);
stringToBinary(key, key_binary);
// 打印明文、密钥和二进制数据
cout << "Plaintext: " << plaintext << endl;
cout << "Key: " << key << endl;
cout << "Plaintext binary: ";
printBinary(plaintext_binary, plaintext_len * 8);
cout << "Key binary: ";
printBinary(key_binary, key_len * 8);
// 创建 DES_KEY
DES_cblock des_key;
memcpy(des_key, key_binary, 8);
DES_key_schedule key_schedule;
DES_set_key_unchecked(&des_key, &key_schedule);
// 加密
unsigned char ciphertext_binary[plaintext_len * 8];
DES_ecb_encrypt((DES_cblock *)plaintext_binary, (DES_cblock *)ciphertext_binary, &key_schedule, DES_ENCRYPT);
// 打印密文和二进制数据
cout << "Ciphertext binary: ";
printBinary(ciphertext_binary, plaintext_len * 8);
// 将密文转成字符串
char ciphertext[plaintext_len + 1];
binaryToString(ciphertext_binary, ciphertext);
ciphertext[plaintext_len] = 0;
// 打印密钥和解密后的明文
cout << "Key: " << key << endl;
cout << "Plaintext after decryption: " << endl;
// 解密
unsigned char decrypted_binary[plaintext_len * 8];
DES_ecb_encrypt((DES_cblock *)ciphertext_binary, (DES_cblock *)decrypted_binary, &key_schedule, DES_DECRYPT);
// 将解密后的明文转成字符串
char decrypted[plaintext_len + 1];
binaryToString(decrypted_binary, decrypted);
decrypted[plaintext_len] = 0;
// 打印解密后的明文
cout << decrypted << endl;
return 0;
}
```
您可以将 `plaintext` 和 `key` 修改为您需要的值,然后编译并运行程序。程序会输出明文、密钥、加密后的密文、密钥和解密后的明文。
阅读全文
相关推荐
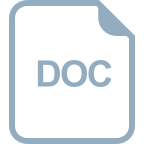
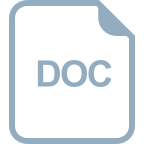
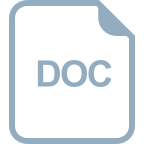


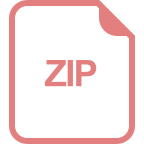
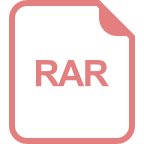
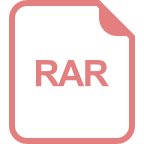
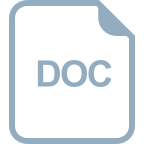
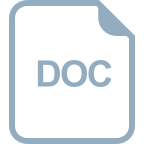
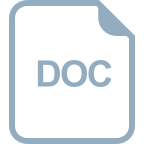
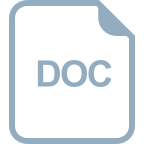


