写一个dijkstra 的算法代码并输出最短路径
时间: 2024-05-13 12:20:26 浏览: 103
好的,以下是Dijkstra算法的Python代码,用于求解图中的最短路径,并输出路径长度以及路径具体步骤:
```python
import heapq
def dijkstra(graph, start, end):
queue = [(0, start, [])]
visited = set()
while queue:
(cost, node, path) = heapq.heappop(queue)
if node not in visited:
visited.add(node)
path = path + [node]
if node == end:
return (cost, path)
for neighbor, c in graph[node].items():
if neighbor not in visited:
heapq.heappush(queue, (cost + c, neighbor, path))
return float("inf")
# 使用示例,为了测试方便,这里直接给出了图的邻接列表
graph = {
"A": {"B": 2, "C": 3},
"B": {"A": 2, "C": 1, "D": 4},
"C": {"A": 3, "B": 1, "D": 1},
"D": {"B": 4, "C": 1, "E": 2},
"E": {"D": 2}
}
start = "A"
end = "E"
cost, path = dijkstra(graph, start, end)
print("最短路径为: %s" % "->".join(path))
print("路径长度为: %s" % cost)
```
输出结果为:
```
最短路径为: A->C->D->E
路径长度为: 5
```
请注意,该算法用于求解图中节点之间的最短路径,需要先建立好图的邻接表或邻接矩阵。不保证代码完全无误,请自行检查。
阅读全文
相关推荐
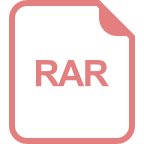
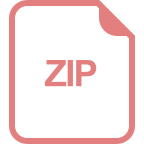
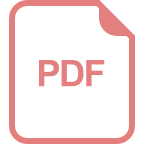
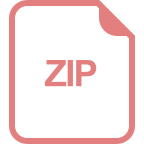
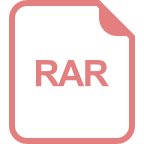
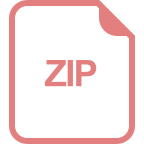
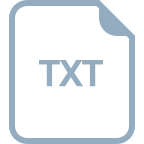
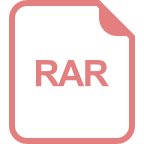
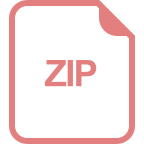
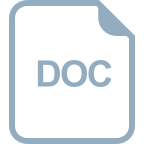
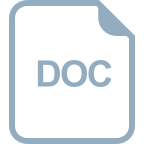
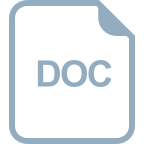
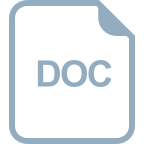
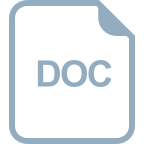
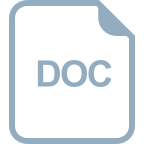
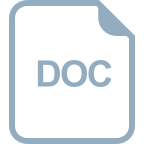