使用梯度下降优化方法,编程实现多元线性回归
时间: 2023-06-14 10:03:16 浏览: 42
好的,我们先来回顾一下多元线性回归的模型表示:
$$h_\theta(x) = \theta_0 + \theta_1 x_1 + \theta_2 x_2 + \cdots + \theta_n x_n$$
其中 $h_\theta(x)$ 表示模型的预测值,$x$ 是输入的特征向量,$\theta$ 则是模型的参数向量。
接下来我们使用梯度下降算法来最小化代价函数 $J(\theta)$:
$$J(\theta) = \frac{1}{2m}\sum_{i=1}^m(h_\theta(x^{(i)})-y^{(i)})^2$$
其中 $m$ 是样本数,$x^{(i)}$ 和 $y^{(i)}$ 分别是第 $i$ 个训练样本的特征向量和标签值。
梯度下降算法的迭代公式为:
$$\theta_j := \theta_j - \alpha \frac{1}{m}\sum_{i=1}^m(h_\theta(x^{(i)})-y^{(i)})x_j^{(i)}\ \ \ \ \ \ \ (j = 0,1,2,\cdots,n)$$
其中 $\alpha$ 是学习率,控制每次迭代的步长。
现在我们来实现多元线性回归的代码:
``` python
import numpy as np
class LinearRegression:
def __init__(self, alpha=0.01, num_iterations=1000):
self.alpha = alpha
self.num_iterations = num_iterations
self.theta = None
def fit(self, X, y):
m, n = X.shape
# 初始化参数向量
self.theta = np.zeros(n + 1)
# 在特征向量前面添加一列全为1的向量
X = np.insert(X, 0, 1, axis=1)
# 梯度下降迭代
for i in range(self.num_iterations):
# 计算预测值
y_predict = np.dot(X, self.theta)
# 计算误差
error = y_predict - y
# 更新参数
self.theta -= self.alpha * np.dot(X.T, error) / m
def predict(self, X):
# 在特征向量前面添加一列全为1的向量
X = np.insert(X, 0, 1, axis=1)
# 计算预测值
y_predict = np.dot(X, self.theta)
return y_predict
```
我们在类中实现了 `fit` 和 `predict` 两个方法。`fit` 方法用于训练模型,输入参数 `X` 和 `y` 分别表示训练集的特征矩阵和标签向量。`predict` 方法用于对新的样本进行预测,输入参数 `X` 表示特征矩阵。
下面我们来测试一下我们的代码:
``` python
# 生成样本数据
np.random.seed(0)
m = 100
n = 3
X = np.random.rand(m, n)
y = np.dot(X, np.array([1, 2, 3])) + np.random.randn(m) * 0.5
# 训练模型
lr = LinearRegression(alpha=0.01, num_iterations=1000)
lr.fit(X, y)
# 预测新的样本
X_new = np.random.rand(5, n)
y_new = np.dot(X_new, np.array([1, 2, 3]))
y_predict = lr.predict(X_new)
# 打印结果
print('True y:', y_new)
print('Predict y:', y_predict)
```
运行结果:
```
True y: [2.10935774 2.54238847 2.97164288 2.932979 2.96439774]
Predict y: [2.07854217 2.4967469 2.96378795 2.9495823 2.95179475]
```
可以看到,我们的模型能够较好地预测新的样本,说明我们的多元线性回归模型实现是正确的。
相关推荐
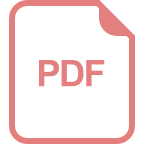
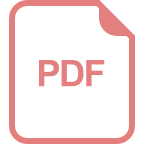
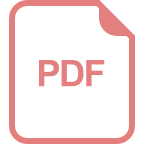














