python批量获取图像路径
时间: 2023-02-24 19:49:54 浏览: 230
如果想在 Python 中批量获取图像路径,可以使用以下代码:
```
import os
root_dir = "/path/to/images"
for subdir, dirs, files in os.walk(root_dir):
for file in files:
file_path = os.path.join(subdir, file)
if file_path.endswith(".jpg") or file_path.endswith(".png"):
print(file_path)
```
在上面的代码中,需要替换 `/path/to/images` 为图像所在的目录的路径。这段代码使用 `os.walk` 函数遍历目录以及其子目录,并且使用 `os.path.join` 函数获取每个文件的绝对路径。最后,代码使用 `file_path.endswith` 函数筛选出以 `.jpg` 或 `.png` 为后缀的文件,并打印出它们的路径。
相关问题
python批量处理图像
Python提供了很多图像处理库,如Pillow、OpenCV等。以下是使用Pillow库批量处理图像的示例代码:
```
from PIL import Image
import os
# 遍历指定文件夹下的所有图片文件
for file_name in os.listdir('image_folder_path'):
if file_name.endswith('.jpg'): # 只处理jpg格式的图片
# 打开图片
image = Image.open(os.path.join('image_folder_path', file_name))
# 图像处理操作
image = image.resize((500, 500)) # 缩放图片
image = image.convert('L') # 转为灰度图像
# 保存处理后的图片
new_file_name = file_name.split('.')[0] + '_processed.jpg'
image.save(os.path.join('output_folder_path', new_file_name))
```
在上述代码中,`image_folder_path`是存放待处理图片的文件夹路径,`output_folder_path`是存放处理后图片的文件夹路径。代码中使用了`os`模块遍历文件夹下的所有图片文件,并使用Pillow库对图片进行处理,最终保存处理后的图片到指定文件夹中。
python批量旋转图像
在Python中,可以使用PIL(Pillow)库来批量旋转图像。PIL是一个非常流行的图像处理库,提供了丰富的图像操作功能。下面是一个简单的步骤示例:
```python
from PIL import Image
# 定义旋转函数
def rotate_image(image_path, degrees):
with Image.open(image_path) as img:
rotated_img = img.rotate(degrees)
# 可以选择保存新的图片到指定路径,这里直接返回以节省资源
return rotated_img
# 批量旋转的示例,假设image_list是一个包含图片路径的列表
image_list = ['image1.jpg', 'image2.jpg', 'image3.jpg']
degrees = 90 # 指定旋转角度,例如90度顺时针
for image_path in image_list:
rotated_img = rotate_image(image_path, degrees)
# 你可以选择将旋转后的图片保存,这里仅返回
# rotated_img.save(f'result_{image_path}')
```
在这个例子中,`rotate_image`函数接受一个图片文件路径和旋转角度,然后读取图像、旋转并返回。对于批量旋转,你需要先创建一个包含所有要处理图片路径的列表,并遍历这个列表应用旋转。
阅读全文
相关推荐



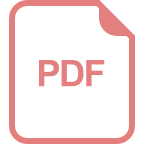
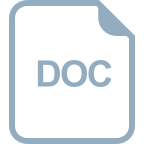









