nano tensorrt yolov7 模型实时摄像头
时间: 2023-10-23 13:11:32 浏览: 139
要在实时摄像头中使用 YOLOv7 模型,您可以使用以下步骤:
1. 下载 YOLOv7 模型的权重和配置文件。您可以在 https://github.com/WongKinYiu/yolov7 中找到这些文件。
2. 安装 TensorRT 和 OpenCV。
3. 在 Python 中加载模型权重和配置文件,将模型转换为 TensorRT 格式,并创建一个 TensorRT 引擎。
4. 使用 OpenCV 读取实时摄像头输入,并将每一帧图像转换为模型输入所需的格式。
5. 将模型输入提供给 TensorRT 引擎,获取模型输出。
6. 处理模型输出并在图像上绘制检测框。
7. 将处理后的图像显示在屏幕上。
以下是一个示例代码,可以在实时摄像头中使用 YOLOv7 模型进行目标检测:
```python
import cv2
import numpy as np
import tensorrt as trt
# 加载模型权重和配置文件
model_weights = 'yolov7.weights'
model_config = 'yolov7.cfg'
# 定义输入和输出节点名称
input_node = 'data'
output_node = ['layer82', 'layer94', 'layer106']
# 定义TensorRT引擎
def build_engine(model_weights, model_config):
with trt.Builder(TRT_LOGGER) as builder, builder.create_network() as network, trt.UffParser() as parser:
builder.max_batch_size = 1
builder.max_workspace_size = 1 << 30
builder.fp16_mode = True
builder.strict_type_constraints = True
# 使用 UFFParser 加载模型
parser.register_input(input_node, (3, 416, 416))
parser.register_output(output_node)
parser.parse(model_config, network)
# 创建TensorRT引擎
engine = builder.build_cuda_engine(network)
return engine
# 创建TensorRT引擎
TRT_LOGGER = trt.Logger(trt.Logger.WARNING)
engine = build_engine(model_weights, model_config)
# 定义模型输入和输出大小
input_size = 416
output_shapes = [(1, 255, 13, 13), (1, 255, 26, 26), (1, 255, 52, 52)]
# 定义预处理函数
def preprocess(img):
img = cv2.resize(img, (input_size, input_size))
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
img = img.astype(np.float32) / 255.0
img = np.transpose(img, (2, 0, 1))
img = np.expand_dims(img, axis=0)
return img
# 定义后处理函数
def postprocess(output, confidence_threshold, nms_threshold):
boxes = []
for i in range(len(output)):
output_i = output[i].reshape((-1, 85))
confidences = output_i[:, 4]
class_ids = output_i[:, 5:].argmax(axis=-1)
mask = confidences > confidence_threshold
confidences = confidences[mask]
class_ids = class_ids[mask]
boxes_i = output_i[mask, :4]
boxes_i[:, 0] -= boxes_i[:, 2] / 2.0
boxes_i[:, 1] -= boxes_i[:, 3] / 2.0
boxes_i[:, 2] += boxes_i[:, 0]
boxes_i[:, 3] += boxes_i[:, 1]
boxes_i = boxes_i.astype(np.int32)
boxes.append((boxes_i, confidences, class_ids))
detections = []
for i in range(len(boxes)):
boxes_i, confidences_i, class_ids_i = boxes[i]
indices = cv2.dnn.NMSBoxes(boxes_i, confidences_i, confidence_threshold, nms_threshold)
for j in indices:
detections.append((boxes_i[j[0]], confidences_i[j[0]], class_ids_i[j[0]]))
return detections
# 打开摄像头
cap = cv2.VideoCapture(0)
# 处理每一帧图像
while True:
ret, frame = cap.read()
if not ret:
break
# 预处理图像
img = preprocess(frame)
# 在TensorRT引擎中执行推理
inputs, outputs, bindings = trt_common.allocate_buffers(engine)
inputs[0].host = img
trt_outputs = trt_common.do_inference(engine, bindings=bindings, inputs=inputs, outputs=outputs)
# 后处理输出
detections = postprocess(trt_outputs, confidence_threshold=0.5, nms_threshold=0.4)
# 在图像上绘制检测框
for box, confidence, class_id in detections:
x1, y1, x2, y2 = box
cv2.rectangle(frame, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.putText(frame, f'{class_id}', (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示图像
cv2.imshow('frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
该代码使用 TensorRT 加速 YOLOv7 模型的推理,并在摄像头输入中实时检测目标。您可以根据自己的需要修改代码中的参数和超参数,以获得更好的检测效果。
阅读全文
相关推荐
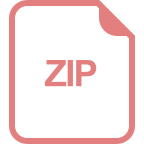

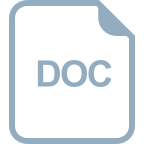
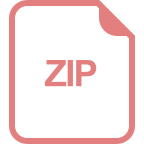
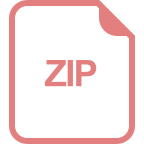
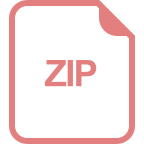
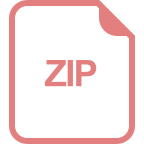
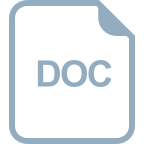
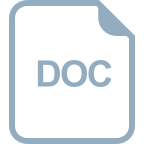
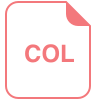
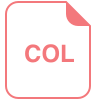
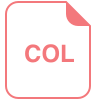
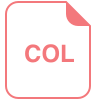
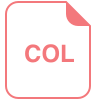


